How to Safely Update the UI from a Thread in Android Timer?
Android Thread for a Timer
Problem
The provided code creates a timer that counts down from 5 minutes to 0:00, but it does not work as expected. The issue lies in updating the UI from within a thread other than the UI thread.
Solution
When working with threads in Android, it is essential to avoid updating the UI from non-UI threads. The following are three alternative approaches to creating a timer that counts down while ensuring UI updates are performed on the UI thread:
1. CountDownTimer
Example:
<code class="java">public class MainActivity extends Activity { private TextView timer1; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.main); timer1 = findViewById(R.id.timer1); startTimer(5 * 60 * 1000); // 5 minutes in milliseconds } private void startTimer(long time) { CountDownTimer timer = new CountDownTimer(time, 1000) { @Override public void onTick(long millisUntilFinished) { int minutes = (int) (millisUntilFinished / (1000 * 60)); int seconds = (int) (millisUntilFinished / 1000) % 60; timer1.setText(String.format("%02d:%02d", minutes, seconds)); } @Override public void onFinish() { timer1.setText("00:00"); } }; timer.start(); } }</code>
2. Handler
Example:
<code class="java">public class MainActivity extends Activity { private Handler mHandler; private Runnable mRunnable; private int timeleft = 5 * 60; // 5 minutes @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.main); mHandler = new Handler(); mRunnable = new Runnable() { @Override public void run() { if (timeleft >= 0) { int minutes = timeleft / 60; int seconds = timeleft % 60; timer1.setText(String.format("%02d:%02d", minutes, seconds)); timeleft--; } else { // Stop the timer mHandler.removeCallbacks(mRunnable); } mHandler.postDelayed(mRunnable, 1000); } }; mRunnable.run(); } }</code>
3. Timer
Example:
<code class="java">public class MainActivity extends Activity { private Timer timer; private TimerTask timerTask; private int timeleft = 5 * 60; // 5 minutes @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.main); timer = new Timer(); timerTask = new TimerTask() { @Override public void run() { runOnUiThread(new Runnable() { @Override public void run() { if (timeleft >= 0) { int minutes = timeleft / 60; int seconds = timeleft % 60; timer1.setText(String.format("%02d:%02d", minutes, seconds)); timeleft--; } else { // Cancel the timer timer.cancel(); } } }); } }; timer.scheduleAtFixedRate(timerTask, 1000, 1000); } }</code>
The above is the detailed content of How to Safely Update the UI from a Thread in Android Timer?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
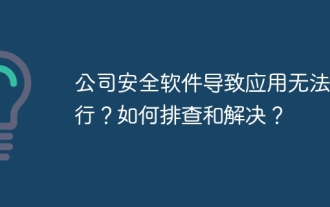
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
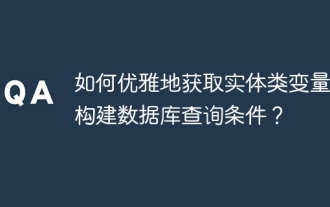
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
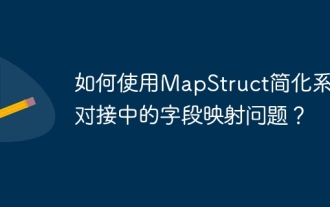
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
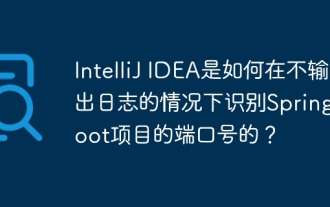
Start Spring using IntelliJIDEAUltimate version...
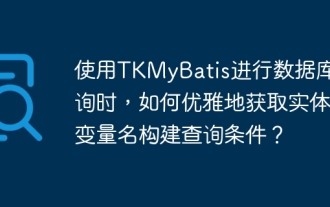
When using TKMyBatis for database queries, how to gracefully get entity class variable names to build query conditions is a common problem. This article will pin...
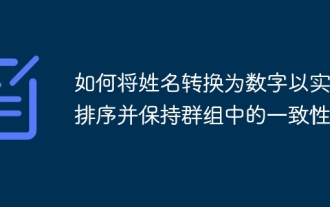
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
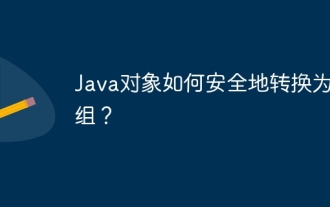
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
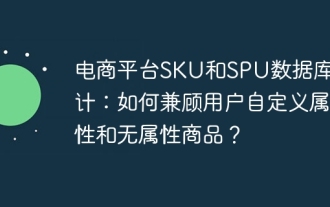
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
