


To Name or Not to Name: When Should You Assign Names to Lambdas in Python?
Should You Name Lambdas in Python?
Lambda expressions provide a concise and convenient way to define anonymous functions in Python. However, it is often tempting to assign names to lambdas within functions for code reuse and specific functionality.
Critique of Naming Lambdas
While this practice may seem convenient, it is generally considered non-Pythonic and goes against the recommendation of PEP8. As PEP8 states:
"Always use a def statement instead of an assignment statement that binds a lambda expression directly to an identifier."
The rationale behind this recommendation is that defining a lambda as a function:
- Provides a clear and explicit name: Unlike named lambdas, def statements explicitly associate the function with a meaningful identifier.
- Helps with traceback and string representations: Named functions display their specific name in error messages and string representations, providing better readability.
- Eliminates the primary benefit of lambdas: The ability to embed anonymous functions within larger expressions is redundant when you assign a variable to a lambda.
Example
Consider the example function provided in the question:
def fcn_operating_on_arrays(array0, array1): indexer = lambda a0, a1, idx: a0[idx] + a1[idx] # ... indexed = indexer(array0, array1, indices) # ...
Instead of using a named lambda, it is more Pythonic to define a separate, explicitly named function:
def index_array(a0, a1, idx): return a0[idx] + a1[idx] def fcn_operating_on_arrays(array0, array1): # ... indexed = index_array(array0, array1, indices) # ...
Conclusion
While assigning names to lambdas may seem useful in certain situations, it is not recommended. Instead, follow the Pythonic principle of using explicit function definitions to maintain clarity, readability, and adherence to best practices.
The above is the detailed content of To Name or Not to Name: When Should You Assign Names to Lambdas in Python?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










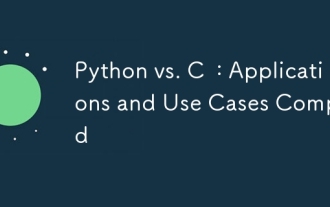
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
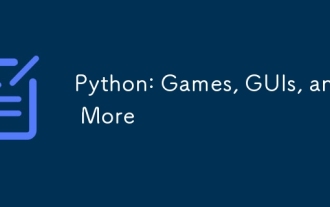
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
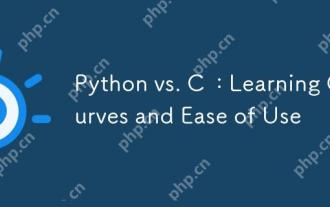
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
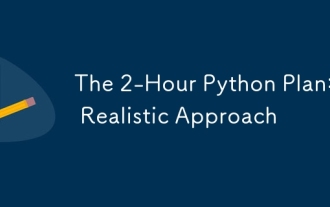
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
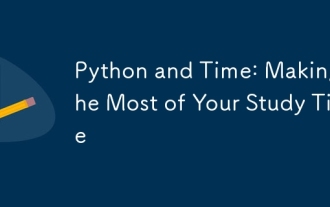
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
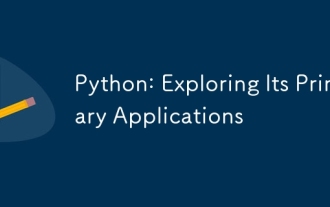
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
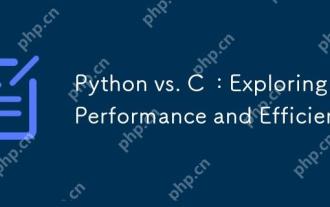
Python is better than C in development efficiency, but C is higher in execution performance. 1. Python's concise syntax and rich libraries improve development efficiency. 2.C's compilation-type characteristics and hardware control improve execution performance. When making a choice, you need to weigh the development speed and execution efficiency based on project needs.
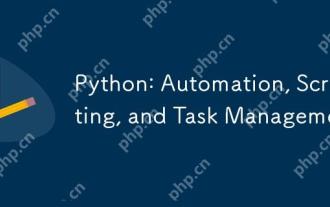
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
