


How Can I Properly Initialize Complex Static Variables in PHP?
Nov 27, 2024 pm 05:44 PMInitializing Static Variables in PHP
When initializing static variables in PHP, you may encounter syntax errors with non-trivial expressions in the initializer, as seen in the code snippet below:
private static $dates = array( 'start' => mktime( 0, 0, 0, 7, 30, 2009), // Start date 'end' => mktime( 0, 0, 0, 8, 2, 2009), // End date 'close' => mktime(23, 59, 59, 7, 20, 2009), // Date when registration closes 'early' => mktime( 0, 0, 0, 3, 19, 2009), // Date when early bird discount ends );
Workaround:
One solution is to avoid complex expressions in the initializer and instead assign the values after defining the class:
class Foo { static $bar; } Foo::$bar = array(…);
Another option is to use a static function to initialize the variable:
class Foo { private static $bar; static function init() { self::$bar = array(…); } } Foo::init();
PHP 5.6 Improvement:
PHP 5.6 supports some expressions in static variable initializers. For example, you can define an abstract class with a private static function to initialize the variable:
abstract class Foo{ private static function bar(){ static $bar = null; if ($bar == null) bar = array(...); return $bar; } /* use where necessary */ self::bar(); }
The above is the detailed content of How Can I Properly Initialize Complex Static Variables in PHP?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
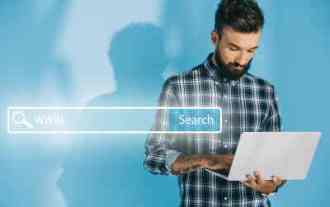
11 Best PHP URL Shortener Scripts (Free and Premium)
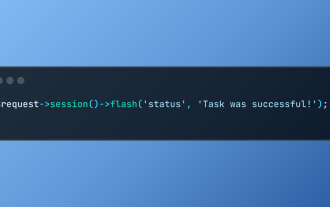
Working with Flash Session Data in Laravel
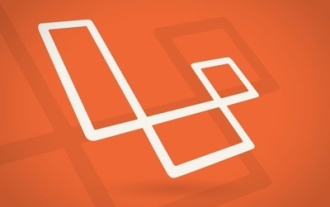
Build a React App With a Laravel Back End: Part 2, React
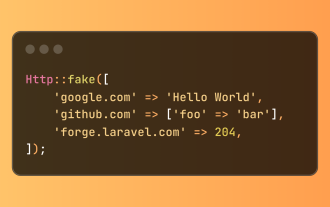
Simplified HTTP Response Mocking in Laravel Tests
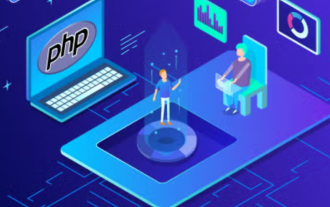
cURL in PHP: How to Use the PHP cURL Extension in REST APIs
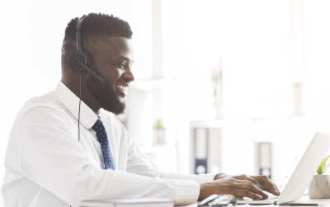
12 Best PHP Chat Scripts on CodeCanyon
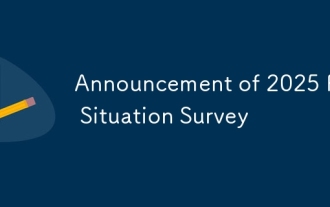
Announcement of 2025 PHP Situation Survey
