


Why Does Go\'s `time.Parse` Fail to Accurately Parse Time Zones, and How Can This Be Fixed?
Understanding Time Zone Parsing in Go
Parsing time zone codes in Go involves converting a string representation of a time zone into a corresponding *time.Location object. However, in certain scenarios, the parsed result may not accurately reflect the desired time zone. This article explores a common issue in time zone parsing and provides solutions.
Problem Formulation
Consider the following code:
package main import ( "fmt" "time" ) func main() { now := time.Now() parseAndPrint(now, "BRT") parseAndPrint(now, "EDT") parseAndPrint(now, "UTC") } func parseAndPrint(now time.Time, timezone string) { test, err := time.Parse("15:04:05 MST", fmt.Sprintf("05:00:00 %s", timezone)) if err != nil { fmt.Println(err) return } test = time.Date( now.Year(), now.Month(), now.Day(), test.Hour(), test.Minute(), test.Second(), test.Nanosecond(), test.Location(), ) fmt.Println(test.UTC()) }
When running this code, the output always shows "[date] 05:00:00 0000 UTC", regardless of the specified time zone. This is because the code is parsing the time in the current location and then setting the time zone explicitly to UTC.
Solution: Using time.Location
To correctly handle time zone parsing, we need to use the *time.Location type. We can load a location from the local timezone database using time.LoadLocation and then parse the time using time.ParseInLocation. Here's the modified code:
package main import ( "fmt" "time" ) func main() { now := time.Now() parseAndPrint(now, "BRT") parseAndPrint(now, "EDT") parseAndPrint(now, "UTC") } func parseAndPrint(now time.Time, timezone string) { location, err := time.LoadLocation(timezone) if err != nil { fmt.Println(err) return } test, err := time.ParseInLocation("15:04:05 MST", "05:00:00", location) if err != nil { fmt.Println(err) return } fmt.Println(test) }
Now, the code will correctly parse the time zone specific time and print the result in the desired time zone format.
The above is the detailed content of Why Does Go\'s `time.Parse` Fail to Accurately Parse Time Zones, and How Can This Be Fixed?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










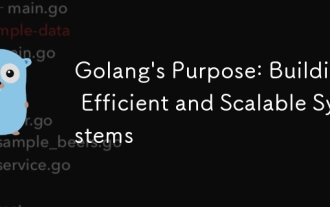
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
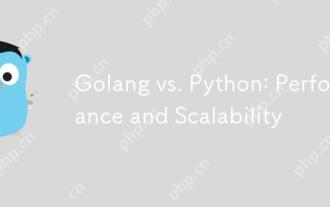
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
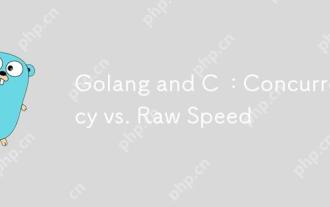
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
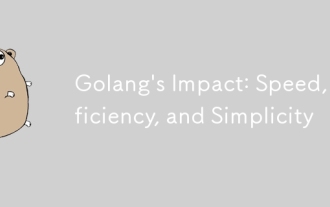
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
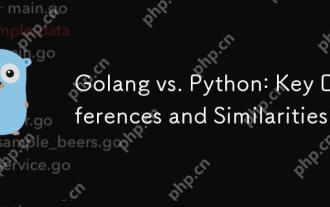
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
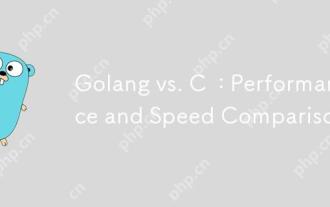
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
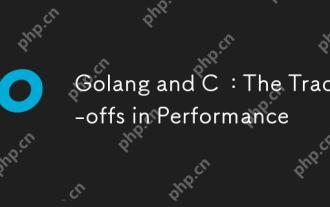
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
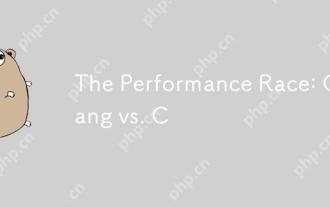
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
