What\'s the Difference Between Bitwise and Logical Operators in Programming?
Nov 27, 2024 pm 05:59 PMClarifying the Differences between Bitwise and Logical Operators
In programming, boolean operators play a crucial role in decision-making and flow control. However, when it comes to bitwise operators (& and |) and logical operators (&& and ||), the distinctions can be confusing.
Understanding Bitwise Operators
Bitwise operators perform operations on individual bits within the binary representation of their inputs. Unlike logical operators, they don't evaluate true or false but instead manipulate the actual bit patterns.
For example, consider the following:
int a = 6; // 110 (binary) int b = 4; // 100 (binary) // Bitwise AND (a & b) int c = a & b; // 110 // & 100 // ----- // 100 (binary) // Bitwise OR (a | b) int d = a | b; // 110 // | 100 // ----- // 110 (binary)
In this case, the bitwise AND (a & b) result in 100 (decimal), which is the common bits set to 1 in both a and b. Conversely, the bitwise OR (a | b) results in 110, which is the bits set to 1 in either a or b.
Contrasting with Logical Operators
Logical operators, on the other hand, operate on boolean values (true or false) and behave as follows:
- Logical AND (a && b): Returns true if both a and b are true, otherwise false.
- Logical OR (a || b): Returns true if either a or b is true, otherwise false.
Key Behavioral Differences
The main difference between bitwise and logical operators is in their evaluation logic:
- Bitwise operators work on bit patterns and perform bit-level operations.
- Logical operators work on boolean values and evaluate truthfulness.
Furthermore, logical operators short-circuit while bitwise operators do not. Short-circuiting means that the evaluation stops as soon as the result is known. This difference becomes important when dealing with potential exceptions or unwanted side effects.
The above is the detailed content of What\'s the Difference Between Bitwise and Logical Operators in Programming?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
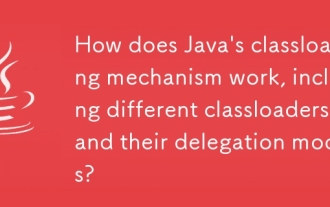
How does Java's classloading mechanism work, including different classloaders and their delegation models?
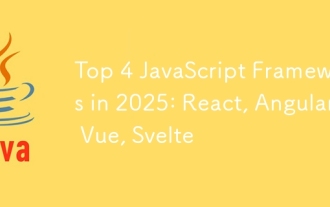
Top 4 JavaScript Frameworks in 2025: React, Angular, Vue, Svelte
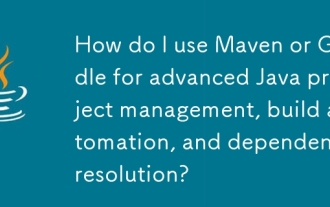
How do I use Maven or Gradle for advanced Java project management, build automation, and dependency resolution?
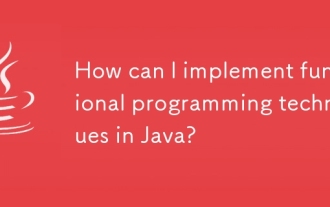
How can I implement functional programming techniques in Java?
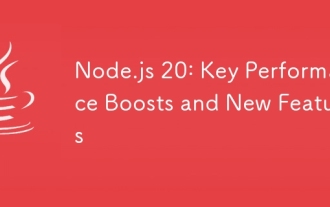
Node.js 20: Key Performance Boosts and New Features
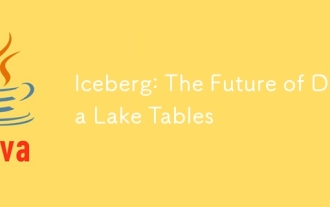
Iceberg: The Future of Data Lake Tables
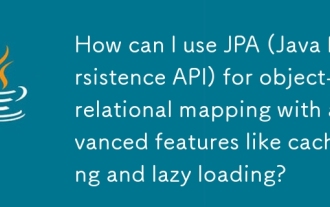
How can I use JPA (Java Persistence API) for object-relational mapping with advanced features like caching and lazy loading?
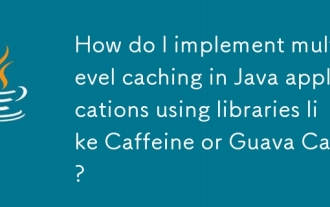
How do I implement multi-level caching in Java applications using libraries like Caffeine or Guava Cache?
