How Can I Trigger CSS Animations Using JavaScript?
Trigger CSS Animations in JavaScript
Triggering CSS animations with plain JavaScript is a simple but effective way to add interactivity to your web pages. This approach is especially useful when you need to target specific animations for a range of events without resorting to jQuery or other frameworks.
Using Event Listeners to Trigger Animations
To trigger an animation using JavaScript, you can use event listeners to listen for specific events that trigger the animation. For example, you can use the scroll event to trigger an animation when a user scrolls the page.
// Add event listener to the window object window.addEventListener('scroll', function() { // Get the element(s) you want to animate var elements = document.querySelectorAll('.my-animation'); // Loop through the elements and start the animation for (var i = 0; i < elements.length; i++) { elements[i].style.animation = "my-animation 2s 2s forward"; } });
Using Class Manipulation
Another method to trigger animations is by adding or removing classes from the element. This is typically done with JavaScript's classList API. For instance, you can add or remove a class called animated to toggle an animation on the fly.
// Get the element(s) you want to animate var elements = document.querySelectorAll('.my-animation'); // Add the "animated" class to start the animation for (var i = 0; i < elements.length; i++) { elements[i].classList.add('animated'); } // Remove the "animated" class to stop the animation for (var i = 0; i < elements.length; i++) { elements[i].classList.remove('animated'); }
By combining event listeners and class manipulation, you can trigger CSS animations in JavaScript and control the timing and behavior of these animations in response to user interactions or other events.
The above is the detailed content of How Can I Trigger CSS Animations Using JavaScript?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










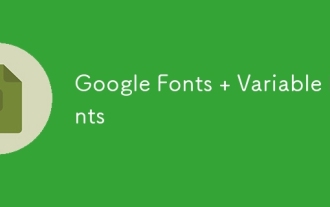
I see Google Fonts rolled out a new design (Tweet). Compared to the last big redesign, this feels much more iterative. I can barely tell the difference
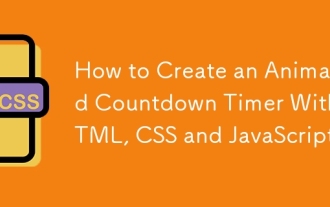
Have you ever needed a countdown timer on a project? For something like that, it might be natural to reach for a plugin, but it’s actually a lot more
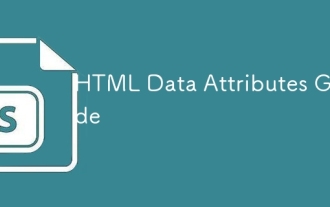
Everything you ever wanted to know about data attributes in HTML, CSS, and JavaScript.
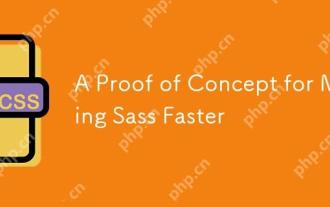
At the start of a new project, Sass compilation happens in the blink of an eye. This feels great, especially when it’s paired with Browsersync, which reloads
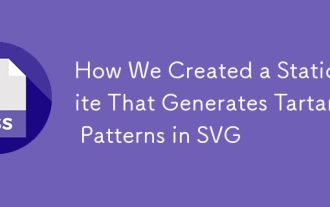
Tartan is a patterned cloth that’s typically associated with Scotland, particularly their fashionable kilts. On tartanify.com, we gathered over 5,000 tartan
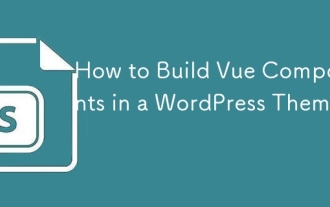
The inline-template directive allows us to build rich Vue components as a progressive enhancement over existing WordPress markup.
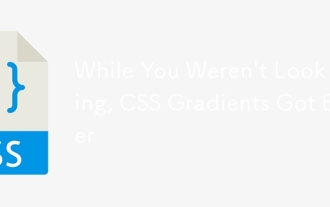
One thing that caught my eye on the list of features for Lea Verou's conic-gradient() polyfill was the last item:
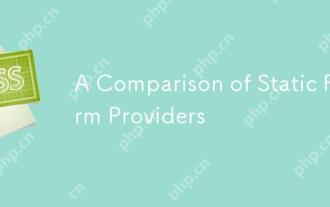
Let’s attempt to coin a term here: "Static Form Provider." You bring your HTML
