


How Can I Convert Seconds to Hours, Minutes, and Seconds in Python?
Nov 27, 2024 pm 09:59 PMWorking with Time Conversions in Python: A Guide to Converting Seconds to Hours, Minutes, and Seconds
In programming, it's often necessary to deal with time values in various formats. When dealing with seconds, you may encounter a need to convert them into a more readable and organized format like hours, minutes, and seconds. Python provides an easy and efficient solution for this conversion.
Converting Seconds to Hours, Minutes, and Seconds
To convert seconds into a format like "hours:minutes:seconds," you can utilize Python's datetime.timedelta function. This function takes the number of seconds as an argument and returns a timedelta object. This timedelta object represents the duration of time in a structured layout.
Here's an example:
>>> import datetime >>> str(datetime.timedelta(seconds=666)) '0:11:06'
In this example, we convert 666 seconds to a time string in the format of "0:11:06." The timedelta object automatically handles the conversion and formatting.
Customizing the Output Format
The datetime.timedelta function provides flexibility in customizing the output format. You can access the hours, minutes, and seconds attributes individually if you want more control over the output. For instance, the following code retrieves the individual components of the timedelta object:
>>> delta = datetime.timedelta(seconds=666) >>> print("Hours:", delta.seconds // 3600) >>> print("Minutes:", delta.seconds % 3600 // 60) >>> print("Seconds:", delta.seconds % 60)
This code prints the breakdown of 666 seconds into its corresponding hours, minutes, and seconds.
Conclusion
Converting seconds to hours, minutes, and seconds in Python is a straightforward task with the help of the datetime.timedelta function. By leveraging this function, you can efficiently convert time values into a more readable and manageable format, making your code more accurate and user-friendly.
The above is the detailed content of How Can I Convert Seconds to Hours, Minutes, and Seconds in Python?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
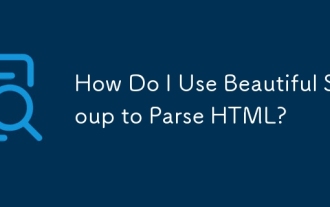
How Do I Use Beautiful Soup to Parse HTML?
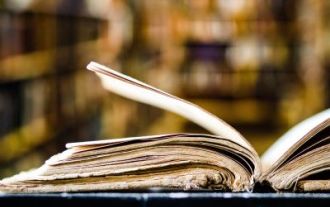
How to Use Python to Find the Zipf Distribution of a Text File
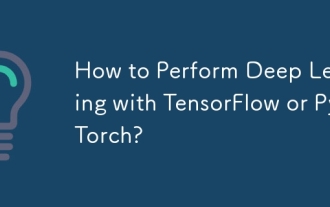
How to Perform Deep Learning with TensorFlow or PyTorch?
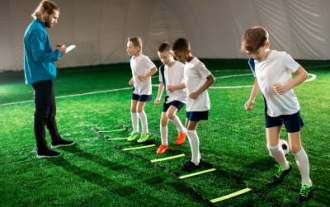
Introduction to Parallel and Concurrent Programming in Python
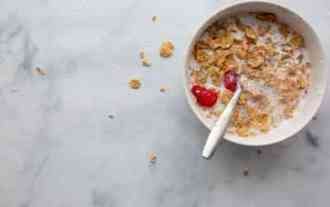
Serialization and Deserialization of Python Objects: Part 1
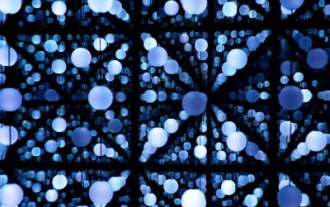
How to Implement Your Own Data Structure in Python
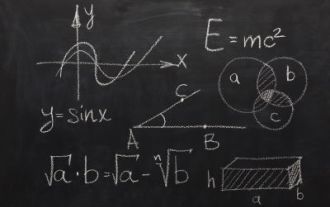
Mathematical Modules in Python: Statistics
