


How Can I Use Regular Expressions for URL Routing in Go\'s `http.HandleFunc()`?
Using Regular Expressions in URL Handling in Golang
In Golang, the http.HandleFunc() method allows you to register URL patterns for HTTP request handling. However, this method does not support using regular expressions for pattern matching. This raises the question of how to use regular expressions to determine appropriate function processing based on the URL pattern.
The standard library's http.HandleFunc() does not support regular expressions for URL matching. Instead, it can only match fixed, rooted paths (e.g., "/favicon.ico") or rooted subtrees (e.g., "/images/"). While longer patterns take precedence over shorter ones, the options are limited.
To overcome this limitation and use regular expressions for URL patterns, you can override the root URL pattern with a more generic pattern. Inside the handler function, you can then perform further regular expression matching and routing based on the specific URL path.
Using Regexp to Route Requests
The following code demonstrates how to use regular expressions for URL routing:
import ( "fmt" "net/http" "regexp" ) func main() { http.HandleFunc("/", route) http.ListenAndServe(":8080", nil) } var rNum = regexp.MustCompile(`\d`) // Has digit(s) var rAbc = regexp.MustCompile(`abc`) // Contains "abc" func route(w http.ResponseWriter, r *http.Request) { switch { case rNum.MatchString(r.URL.Path): digits(w, r) case rAbc.MatchString(r.URL.Path): abc(w, r) default: w.Write([]byte("Unknown Pattern")) } } func digits(w http.ResponseWriter, r *http.Request) { w.Write([]byte("Has digits")) } func abc(w http.ResponseWriter, r *http.Request) { w.Write([]byte("Has abc")) }
In this example, the main function registers the root URL ("/") as a wildcard pattern. Inside the route() function, it uses regular expressions (rNum and rAbc) to match two specific URL patterns: paths with at least one digit (e.g., "/123") and paths containing the string "abc" (e.g., "/abcde"). If a request matches either pattern, the appropriate function (e.g., digits() or abc()) is executed. Otherwise, a default message is displayed.
Alternative Approaches
In addition to the above approach, you can also use external libraries like Gorilla MUX, which provides a more flexible routing mechanism with support for regular expressions.
The above is the detailed content of How Can I Use Regular Expressions for URL Routing in Go\'s `http.HandleFunc()`?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


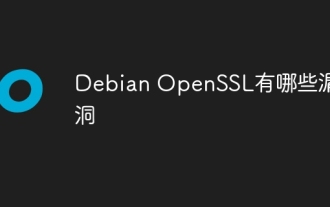
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
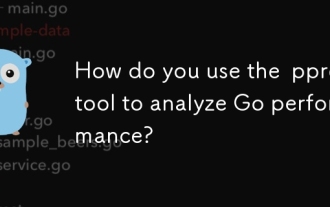
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
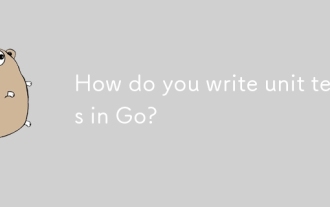
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
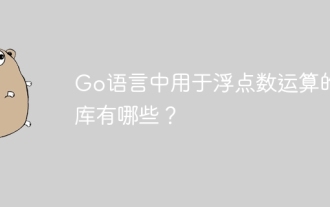
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
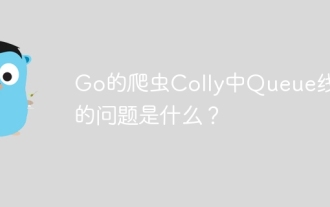
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
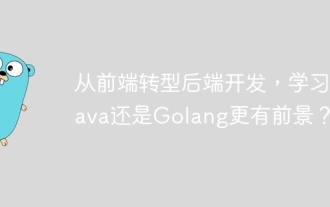
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
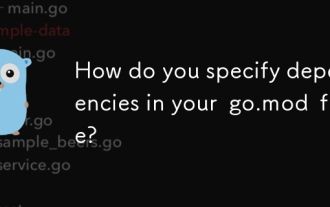
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.
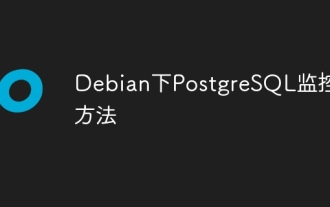
This article introduces a variety of methods and tools to monitor PostgreSQL databases under the Debian system, helping you to fully grasp database performance monitoring. 1. Use PostgreSQL to build-in monitoring view PostgreSQL itself provides multiple views for monitoring database activities: pg_stat_activity: displays database activities in real time, including connections, queries, transactions and other information. pg_stat_replication: Monitors replication status, especially suitable for stream replication clusters. pg_stat_database: Provides database statistics, such as database size, transaction commit/rollback times and other key indicators. 2. Use log analysis tool pgBadg
