How Can I Use Default Values with Pass-by-Reference Parameters in C ?
Passing Parameters by Reference with Default Values in C
In C , function parameters can be passed both by value and by reference. When passing by value, a copy of the parameter's value is made and this copy is passed to the function. When passing by reference, the function operates directly on the memory address of the parameter.
When passing a parameter by reference, it is not possible to specify a default value in the same way as you can for parameters passed by value. This is because a default value is initialized when the function is defined, but a reference parameter is only initialized when the function is called with arguments passed in.
To illustrate, consider the following function declaration:
virtual const ULONG Write(ULONG &State = 0, bool sequence = true);
Here, the "State" parameter is declared as a reference to a ULONG and the "sequence" parameter is declared as a boolean. However, assigning a default value to "State" ("= 0") causes an error:
error C2440: 'default argument' : cannot convert from 'const int' to 'unsigned long &' A reference that is not to 'const' cannot be bound to a non-lvalue
This error occurs because a non-const reference cannot be bound to a non-lvalue (i.e., a temporary expression). However, it is possible to specify a default value for a const reference:
virtual const ULONG Write(const ULONG &State = 0, bool sequence = true);
In this case, the "State" parameter is a const reference to a ULONG and the default value ("= 0") is a const lvalue. This allows the function to be called without having to specify a value for "State".
One exception to this rule is when using a static variable as the default value for a non-const reference:
static int AVAL = 1; void f(int &x = AVAL) { // stuff } int main() { f(); // equivalent to f(AVAL); }
In this example, the "x" parameter is a non-const reference to an int and the default value ("AVAL") is a static variable. This allows the function to be called without having to specify a value for "x". However, this technique is of limited practical use.
The above is the detailed content of How Can I Use Default Values with Pass-by-Reference Parameters in C ?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










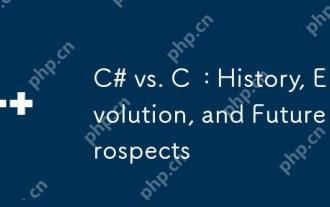
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
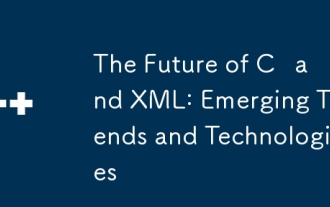
The future development trends of C and XML are: 1) C will introduce new features such as modules, concepts and coroutines through the C 20 and C 23 standards to improve programming efficiency and security; 2) XML will continue to occupy an important position in data exchange and configuration files, but will face the challenges of JSON and YAML, and will develop in a more concise and easy-to-parse direction, such as the improvements of XMLSchema1.1 and XPath3.1.
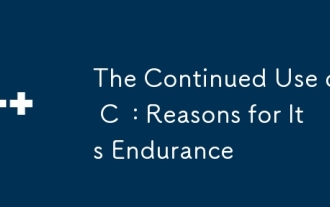
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.
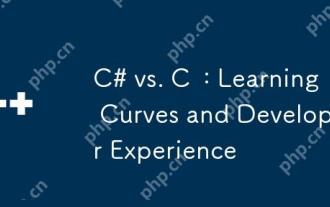
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
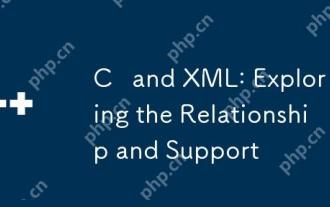
C interacts with XML through third-party libraries (such as TinyXML, Pugixml, Xerces-C). 1) Use the library to parse XML files and convert them into C-processable data structures. 2) When generating XML, convert the C data structure to XML format. 3) In practical applications, XML is often used for configuration files and data exchange to improve development efficiency.
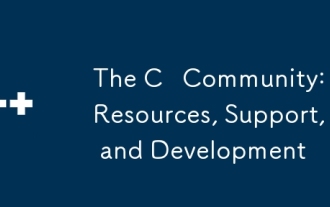
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
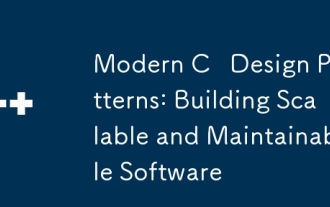
The modern C design model uses new features of C 11 and beyond to help build more flexible and efficient software. 1) Use lambda expressions and std::function to simplify observer pattern. 2) Optimize performance through mobile semantics and perfect forwarding. 3) Intelligent pointers ensure type safety and resource management.
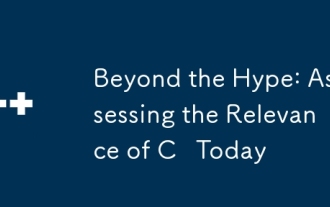
C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
