Why Does My PHP Weekend Date Check Always Return False?
Nov 28, 2024 am 12:42 AMVerifying Weekend Dates in PHP
While attempting to determine if a given date falls within a weekend, you've noticed that your existing function consistently returns 'false.' Let's delve into your code and understand the reason behind this seemingly erroneous behavior.
The challenge lies in the way you're parsing the date. Your code uses the date() function to extract the day of the week as a string (e.g., "Saturday" or "Sunday"), and then compares it to those exact strings. This approach can be problematic due to case sensitivity and the possibility of typos.
Improved Approach:
Instead, you can leverage PHP's built-in date functions to simplify this task. Here are a couple of recommended solutions:
For PHP 5.1 and later:
function isWeekend($date) { return (date('N', strtotime($date)) >= 6); }
This function uses the date('N') function to retrieve the day of the week as an integer (1-7), where 6 and 7 represent Saturday and Sunday, respectively. By comparing the result to 6, you can accurately determine if the date falls on a weekend.
For earlier versions of PHP:
function isWeekend($date) { $weekDay = date('w', strtotime($date)); return ($weekDay == 0 || $weekDay == 6); }
For PHP versions prior to 5.1, you can still obtain the day of the week as an integer using date('w'). In this case, you'll need to check for both Sunday (0) and Saturday (6) as weekend days.
The above is the detailed content of Why Does My PHP Weekend Date Check Always Return False?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
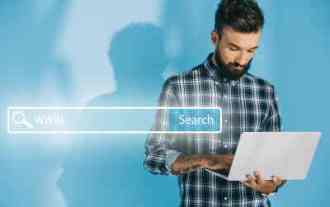
11 Best PHP URL Shortener Scripts (Free and Premium)
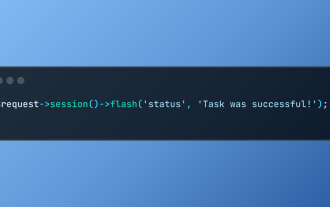
Working with Flash Session Data in Laravel
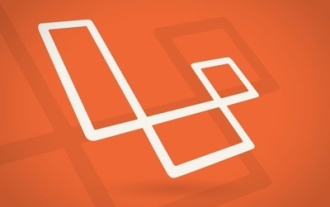
Build a React App With a Laravel Back End: Part 2, React
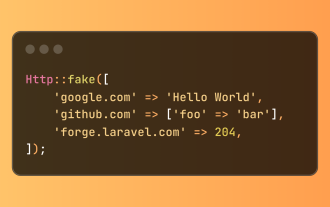
Simplified HTTP Response Mocking in Laravel Tests
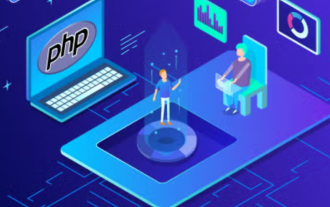
cURL in PHP: How to Use the PHP cURL Extension in REST APIs
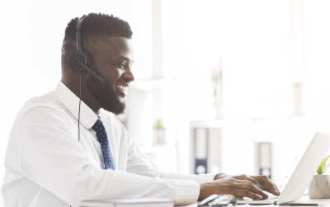
12 Best PHP Chat Scripts on CodeCanyon
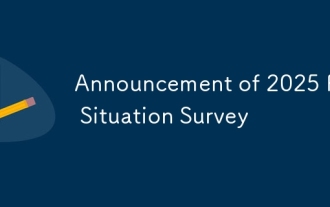
Announcement of 2025 PHP Situation Survey
