


How Can I Efficiently Parse Prometheus Data Using the expfmt Package in Go?
Nov 28, 2024 am 03:07 AMParse Prometheus Data Effectively with expfmt
Parsing Prometheus data can be a challenging task, but with the right tools, it can be a breeze. In this article, we will explore how to parse Prometheus data effectively using the expfmt package.
Prometheus provides an Exposition Format (EBNF syntax) to represent metrics. To decode and encode this format, the Prometheus authors have created the expfmt package, which offers a convenient way to work with Prometheus data in Go.
Sample Input
Let's consider the following Prometheus data as an example:
# HELP net_conntrack_dialer_conn_attempted_total # TYPE net_conntrack_dialer_conn_attempted_total untyped net_conntrack_dialer_conn_attempted_total{dialer_name="federate",instance="localhost:9090",job="prometheus"} 1 1608520832877
Using expfmt
To parse the above data using expfmt, you can follow these steps:
- Import the expfmt package:
import "github.com/prometheus/common/expfmt"
- Create a function to parse the data:
func parseMF(path string) (map[string]*dto.MetricFamily, error) { reader, err := os.Open(path) if err != nil { return nil, err } var parser expfmt.TextParser mf, err := parser.TextToMetricFamilies(reader) if err != nil { return nil, err } return mf, nil }
- Parse the input data:
mf, err := parseMF("/path/to/prometheus_data") if err != nil { log.Fatal(err) }
- Iterate through the parsed data:
for k, v := range mf { fmt.Println("KEY:", k) fmt.Println("VAL:", v) }
Sample Output
Running the above code would produce the following output:
KEY: net_conntrack_dialer_conn_attempted_total VAL: name:"net_conntrack_dialer_conn_attempted_total" type:UNTYPED metric:<label:<name:"dialer_name" value:"federate" > label:<name:"instance" value:"localhost:9090" > label:<name:"job" value:"prometheus" > untyped:<value:1 > timestamp_ms:1608520832877 >
Conclusion
By using the expfmt package, you can effectively parse Prometheus data and gain control over each piece of information to format it in a way that suits your needs. So, next time you need to work with Prometheus data in Go, reach for expfmt to make your development tasks effortless.
The above is the detailed content of How Can I Efficiently Parse Prometheus Data Using the expfmt Package in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
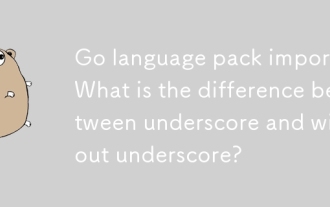
Go language pack import: What is the difference between underscore and without underscore?

How to implement short-term information transfer between pages in the Beego framework?
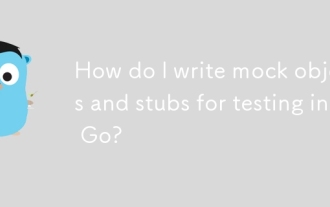
How do I write mock objects and stubs for testing in Go?
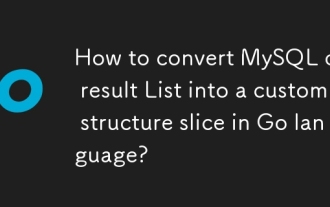
How to convert MySQL query result List into a custom structure slice in Go language?
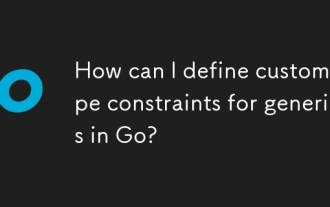
How can I define custom type constraints for generics in Go?
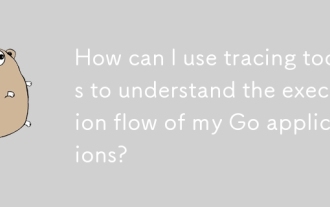
How can I use tracing tools to understand the execution flow of my Go applications?
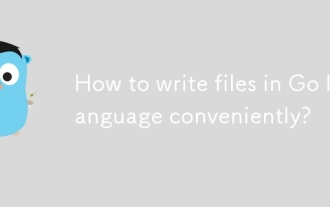
How to write files in Go language conveniently?
