How Can I Create an ExecutorService That Interrupts Tasks After a Timeout?
Timeout Executor Service
Overview
In this context, we are interested in an ExecutorService implementation capable of interrupting tasks that exceed a predefined timeout.
Existing Implementations
One such implementation is TimeoutThreadPoolExecutor, which provides a mechanism to specify a timeout duration for submitted tasks.
Implementation Details
<br>import java.util.List;<br>import java.util.concurrent.*;</p> <p>public class TimeoutThreadPoolExecutor extends ThreadPoolExecutor {</p> <div class="code" style="position:relative; padding:0px; margin:0px;"><pre class="brush:php;toolbar:false">private final long timeout; private final TimeUnit timeoutUnit; // ... (rest of the implementation)
}
Usage
To utilize this executor service, simply create an instance, specifying the desired timeout:
TimeoutThreadPoolExecutor executor = new TimeoutThreadPoolExecutor(..., timeout, TimeUnit.MILLISECONDS);
Then, submit your tasks to the executor as usual. Tasks that exceed the specified timeout will be interrupted.
Alternative Approach
Alternatively, you can employ a ScheduledExecutorService:
ScheduledExecutorService executor = Executors.newScheduledThreadPool(2); Future<?> handler = executor.submit(new Callable() { /* ... */ }); executor.schedule(() -> handler.cancel(true), 10000, TimeUnit.MILLISECONDS);
This strategy ensures that the task is interrupted after 10 seconds.
The above is the detailed content of How Can I Create an ExecutorService That Interrupts Tasks After a Timeout?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










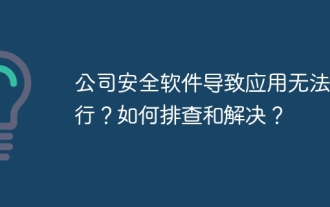
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
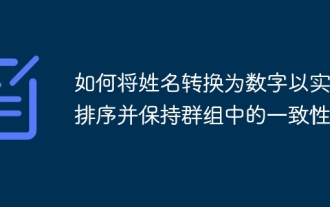
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
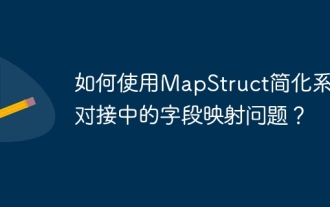
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
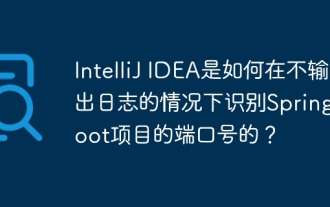
Start Spring using IntelliJIDEAUltimate version...
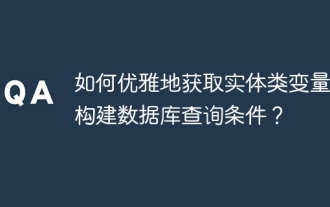
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
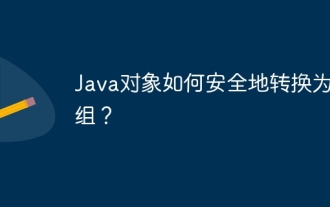
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
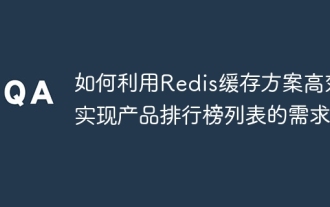
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
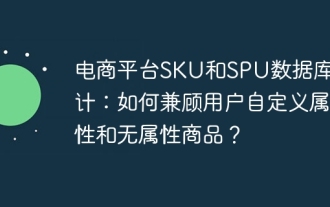
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
