


How to Prevent Memory Leaks When Using Vectors of Object Pointers in C ?
Nov 28, 2024 am 04:37 AMAvoiding Memory Leaks When Using Vectors of Object Pointers
When using vectors of pointers to dynamically allocated objects in C , understanding potential memory leaks and employing proper memory management techniques is crucial.
Vectors automatically manage memory allocations for stored elements, but in the case of vectors of pointers, the memory allocated belongs to the pointers, not the objects they represent. This means that when the vector goes out of scope, its contents (the pointers) will be freed, leaving the allocated object memories dangling and potentially leading to memory leaks.
To prevent this issue, it's important to ensure the deletion of all allocated objects before the vector goes out of scope. One approach is to manually iterate over the vector and call delete on each pointer, but this can be error-prone and inconvenient.
A better solution is to utilize smart pointers, which provide automatic memory management. There are two primary types of smart pointers: unique_ptr and shared_ptr.
std::unique_ptr
std::unique_ptr represents single ownership of a resource. When a unique_ptr goes out of scope, it automatically frees the owned memory. This eliminates the risk of memory leaks and ensures that the corresponding object is deallocated when no longer needed.
Example:
#include <memory> #include <vector> struct base { virtual ~base() {} }; struct derived : base {}; typedef std::vector<std::unique_ptr<base>> container; void foo() { container c; for (int i = 0; i < 100; ++i) c.push_back(std::make_unique<derived>()); } // all automatically freed here int main() { foo(); }
std::shared_ptr
std::shared_ptr is designed for shared ownership. It employs reference counting to track the number of shared pointers pointing to an object. When the last shared_ptr goes out of scope, the owned memory is deallocated, regardless of the number of copies or references outstanding.
Example:
#include <memory> #include <vector> struct base { virtual ~base() {} }; struct derived : base {}; typedef std::vector<std::shared_ptr<base>> container; void foo() { container c; for (int i = 0; i < 100; ++i) c.push_back(std::make_shared<derived>()); } // all automatically freed here int main() { foo(); }
Typically, it's recommended to use std::unique_ptr as it provides more lightweight memory management. However, std::shared_ptr can be useful in situations where shared ownership is desired or when an existing raw pointer needs to be converted into a smart pointer.
Alternatively, boost::ptr_container is a library that provides container classes specifically designed for storing pointers. It automates memory management, similar to the aforementioned smart pointers.
Regardless of the approach used, it's paramount to adopt proper memory management practices and avoid manual explicit deallocation of resources, as this can lead to memory leaks and unpredictable behavior in the application.
The above is the detailed content of How to Prevent Memory Leaks When Using Vectors of Object Pointers in C ?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
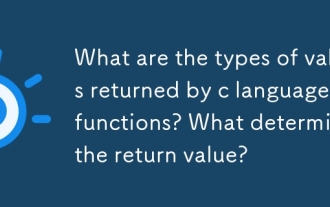
What are the types of values returned by c language functions? What determines the return value?

What are the definitions and calling rules of c language functions and what are the
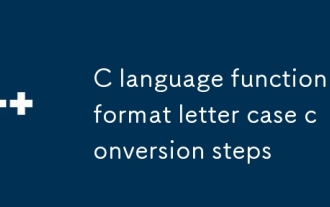
C language function format letter case conversion steps

Where is the return value of the c language function stored in memory?
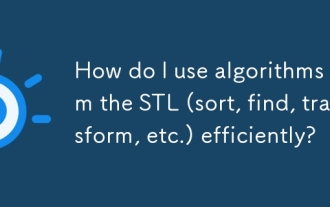
How do I use algorithms from the STL (sort, find, transform, etc.) efficiently?
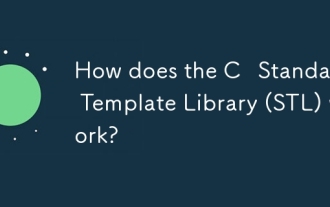
How does the C Standard Template Library (STL) work?
