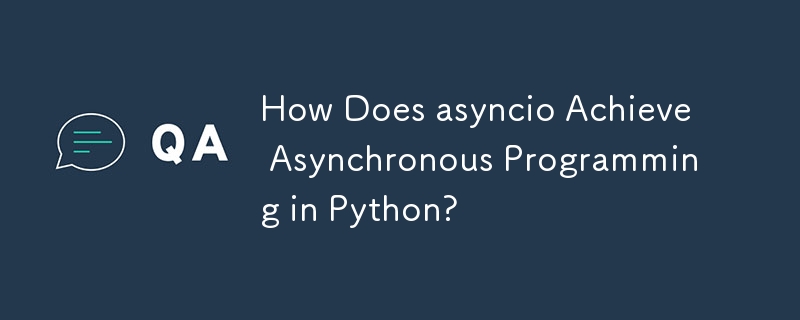
How asyncio Works
Asynchronous programming enables a program to continue running without waiting for input or output (I/O) operations to complete, thereby enhancing performance and responsiveness. One popular Python library that implements asynchronous programming is asyncio.
Fundamentals
Before delving into asyncio's workings, let's consider some fundamental concepts:
-
Generators: Generators are Python objects that yield values, allowing their execution to be paused and resumed.
-
Coroutines: Coroutines are asynchronous generators created using the async def keyword. They pause and resume execution using the await keyword.
asyncio Architecture
At its core, asyncio involves three key components:
-
Tasks: These encapsulate coroutines and communicate with them.
-
Futures: These hold the results or exceptions of asynchronous operations and notify tasks when they become available.
-
Event Loop: This schedules and runs tasks, driving the asynchronous execution.
I/O Implementation
Asynchronous I/O in asyncio is achieved through an event loop and the select() function. Select monitors sockets for data availability or readiness for writing.
- When an I/O operation is performed, asyncio evaluates if data can be immediately processed.
- If the socket requires further action, asyncio registers it with select() and creates a future for it.
- Tasks awaiting these futures pause execution.
- The event loop calls select(), which waits for socket events.
- If an event occurs, the corresponding future is set to done, signifying the availability of data.
- The associated task wakes up and resumes the coroutine, which then reads or writes data.
The Bigger Picture
Asynchronous programming with asyncio allows the event loop to handle I/O operations while other tasks continue running concurrently. This results in a responsive and efficient program that makes optimal use of available resources, particularly in applications involving numerous I/O operations or long-running tasks.
The above is the detailed content of How Does asyncio Achieve Asynchronous Programming in Python?. For more information, please follow other related articles on the PHP Chinese website!