


How Can I Efficiently Merge Go Structs of the Same Type Using JSON Encoding?
Merge Fields of Identical Structures with JSON Encoding
In the realm of Go programming, the task of merging fields from two structures of the same type arises frequently. Consider a scenario where you have a default configuration, such as:
type Config struct { path string id string key string addr string size uint64 } var DefaultConfig = Config{"", "", "", "", 0}
And a configuration loaded from a file, such as:
var FileConfig = Config{"", "file_id", "", "file_addr", 0}
Your objective is to merge these two configurations so that the result possesses the values from both structs, with FileConfig overwriting any values in DefaultConfig. However, FileConfig may not contain all fields.
Originally, you pondered utilizing reflection for this task:
func merge(default *Config, file *Config) (*Config) { b := reflect.ValueOf(default).Elem() o := reflect.ValueOf(file).Elem() for i := 0; i < b.NumField(); i++ { defaultField := b.Field(i) fileField := o.Field(i) if defaultField.Interface() != reflect.Zero(fileField.Type()).Interface() { defaultField.Set(reflect.ValueOf(fileField.Interface())) } } return default }
However, in this instance, reflection is not an optimal solution. A more elegant approach is to leverage the power of the encoding/json package.
The encoding/json package provides a simple mechanism to unmarshal JSON data into a predefined Go struct. Utilizing this technique, you can elegantly merge your configurations:
import ( "encoding/json" "strings" ) const fileContent = `{"id":"file_id","addr":"file_addr","size":100}` func unmarshalConfig(conf *Config, content string) error { return json.NewDecoder(strings.NewReader(content)).Decode(conf) } func mergeConfigs(defConfig *Config, fileConfig *Config) error { if err := unmarshalConfig(defConfig, fileContent); err != nil { return err } for _, v := range fileConfig { defConfig[v.key] = v.value } return nil }
In this solution, the fileConfig is unmarshaled into the default configuration. The encoding/json package handles all the complexities of setting field values, including missing values (which will default to their zero value) and file-specified values that override default values.
By utilizing unmarshaling, you achieve a simple and efficient solution to merge structures of the same type, ensuring that FileConfig fields that are set will take precedence over default values.
The above is the detailed content of How Can I Efficiently Merge Go Structs of the Same Type Using JSON Encoding?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










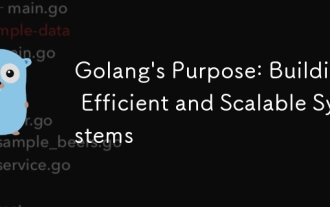
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
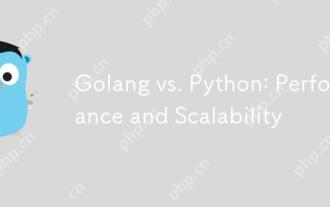
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
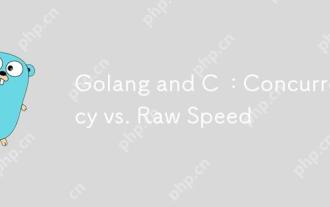
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
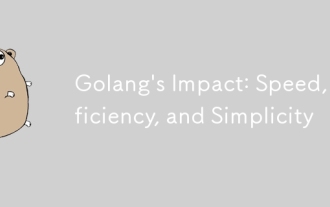
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
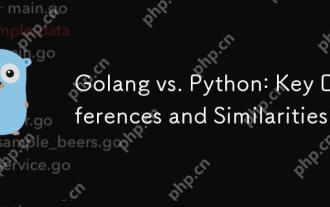
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
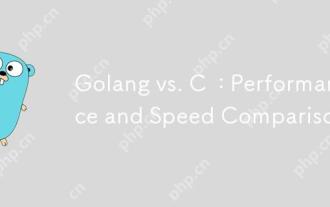
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
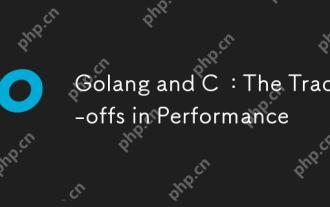
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
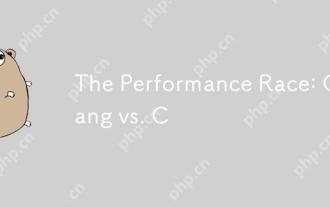
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
