


How to Efficiently Convert Binary to ASCII and Vice Versa in Python?
Convert Binary to ASCII and Vice Versa
Converting between binary and ASCII is essential for encoding and decoding digital data. Here are methods to perform this conversion effectively in Python, addressing the challenges you mentioned:
Converting String to Binary
To convert a string to binary, you can use:
import binascii bin(int(binascii.hexlify('hello'), 16))
This converts the string to a hex representation and then to binary, producing the same output you obtained.
Converting Binary to String
For conversion from binary to string, utilize:
n = int('0b110100001100101011011000110110001101111', 2) binascii.unhexlify('%x' % n)
This transforms the binary string to a hex value and then decodes it to the original string.
Python 3.2 Compatibility
In Python 3.2 and above, you can employ the following methods:
bin(int.from_bytes('hello'.encode(), 'big')) n = int('0b110100001100101011011000110110001101111', 2) n.to_bytes((n.bit_length() + 7) // 8, 'big').decode()
These methods utilize the int.from_bytes() and int.to_bytes() functions, providing an efficient way to handle binary data.
Supporting Unicode Characters (Python 3)
To handle Unicode characters in Python 3, use:
def text_to_bits(text, encoding='utf-8', errors='surrogatepass'): bits = bin(int.from_bytes(text.encode(encoding, errors), 'big'))[2:] return bits.zfill(8 * ((len(bits) + 7) // 8)) def text_from_bits(bits, encoding='utf-8', errors='surrogatepass'): n = int(bits, 2) return n.to_bytes((n.bit_length() + 7) // 8, 'big').decode(encoding, errors) or '<pre class="brush:php;toolbar:false">import binascii def text_to_bits(text, encoding='utf-8', errors='surrogatepass'): bits = bin(int(binascii.hexlify(text.encode(encoding, errors)), 16))[2:] return bits.zfill(8 * ((len(bits) + 7) // 8)) def text_from_bits(bits, encoding='utf-8', errors='surrogatepass'): n = int(bits, 2) return int2bytes(n).decode(encoding, errors) def int2bytes(i): hex_string = '%x' % i n = len(hex_string) return binascii.unhexlify(hex_string.zfill(n + (n & 1)))
These functions encode and decode text with a specified encoding and error handling, ensuring the correct handling of Unicode characters.
Single-Source Python 2/3 Compatible Version
This code is compatible with both Python 2 and 3:
This code handles both Python versions while providing a consistent set of functions for binary and ASCII conversions.
The above is the detailed content of How to Efficiently Convert Binary to ASCII and Vice Versa in Python?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










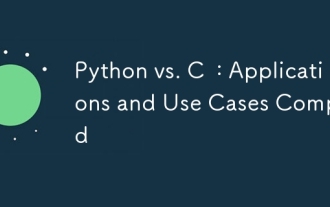
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
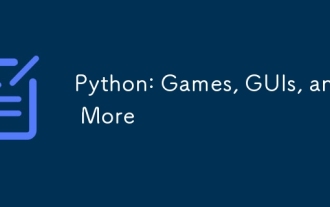
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
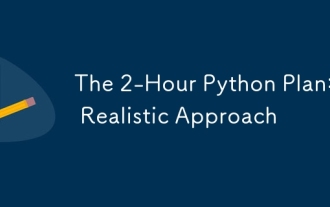
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
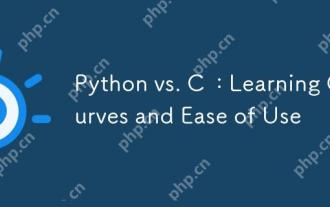
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
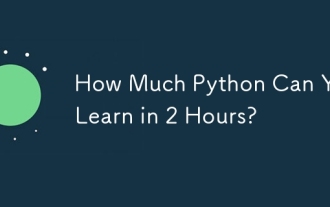
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
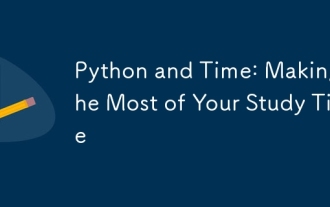
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
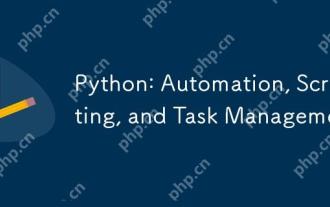
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
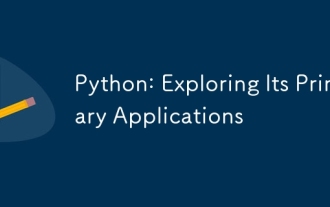
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
