


How Can I Retrieve Return Values from multiprocessing.Process Instances in Python?
Extracting Return Values from Functions in Multiprocessing.Process Instances
The ability to retrieve return values from functions passed to multiprocessing.Process can be a useful feature, particularly when asynchronous task execution is required. Unfortunately, the values are not immediately accessible from the Process object, necessitating an alternative approach.
Understanding Value Storage
Unlike traditional Python functions, those passed to multiprocessing.Process do not have a return value attribute. Instead, the value is stored in a separate location, specifically in a shared memory object. This is because the processes created using multiprocessing run in separate memory spaces, preventing direct access to variables in the main process.
Using Shared Variables for Communication
To access the return value, we need to establish a form of communication between the processes. One effective method is to utilize shared variables. These are objects that allow multiple processes to share and access data simultaneously. In our case, we create a manager object and a shared dictionary using multiprocessing.Manager(). The dictionary acts as the shared variable.
Accessing the Return Value
Within the worker function, we populate the shared dictionary with the desired return value. The main process, after waiting for all tasks to complete, can access and retrieve these values from the shared dictionary. By employing this strategy, we effectively extract the return values without compromising the multiprocessing approach.
Example Implementation
The following example showcases the implementation of shared variables to retrieve return values:
import multiprocessing def worker(procnum, return_dict): """worker function""" print(str(procnum) + " represent!") return_dict[procnum] = procnum if __name__ == "__main__": manager = multiprocessing.Manager() return_dict = manager.dict() jobs = [] for i in range(5): p = multiprocessing.Process(target=worker, args=(i, return_dict)) jobs.append(p) p.start() for proc in jobs: proc.join() print(return_dict.values())
Output:
0 represent! 1 represent! 3 represent! 2 represent! 4 represent! [0, 1, 3, 2, 4]
This approach enables us to retrieve the return values of the worker function and demonstrate the seamless communication between processes in the multiprocessing framework.
The above is the detailed content of How Can I Retrieve Return Values from multiprocessing.Process Instances in Python?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










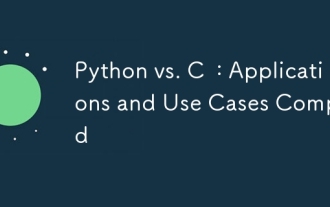
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
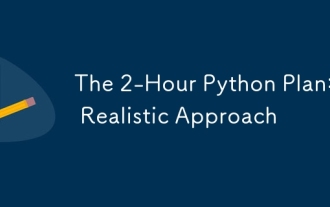
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
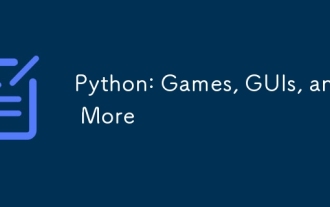
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
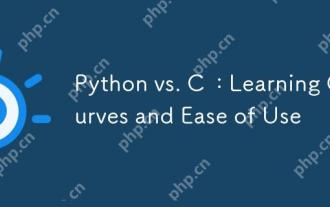
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
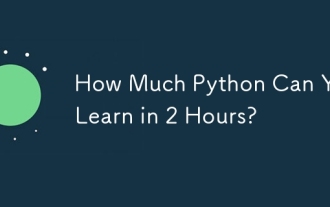
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
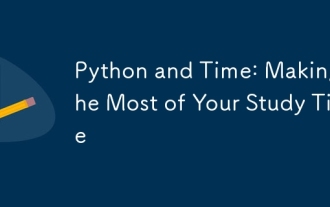
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
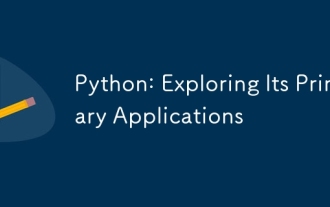
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
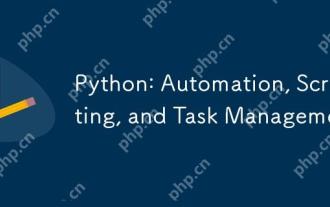
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
