


How Can We Optimize the Sieve of Eratosthenes for Efficient Prime Number Generation in Python?
Sieve of Eratosthenes: Optimizing Prime Number Generation in Python
The Sieve of Eratosthenes is a classic algorithm for finding prime numbers. However, it is crucial to implement it correctly to avoid performance bottlenecks.
Original Implementation
The provided primes_sieve function maintains a list of candidate prime numbers and iteratively removes non-primes by traversing the list and eliminating factors. This approach is inherently inefficient due to the high cost of list manipulation.
Dictionary-Based Optimization
The improved primes_sieve1 function uses a dictionary to store primality flags. While faster than the list-based approach, it still faces challenges. It iterates over the dictionary in an undefined order, causing redundant marking of non-prime factors. Additionally, it converts the final dictionary to a list, incurring unnecessary overhead.
Correct and Efficient Implementation
The correct Sieve of Eratosthenes algorithm utilizes a list of boolean flags to indicate primality. The primes_sieve2 function initializes the flags to True for all numbers and sets the flags for 0 and 1 to False. It iterates through the list, marking non-primes by setting their flags to False.
This approach is efficient because:
- It uses a list instead of a dictionary, avoiding the overhead of key-value operations.
- It marks only prime factors as non-prime, reducing redundant operations.
- It optimizes the marking process by starting at the square of each prime instead of its double.
By implementing the Sieve of Eratosthenes correctly, you can significantly improve the performance of prime number generation, making it suitable even for large input limits like finding primes under 2 million.
The above is the detailed content of How Can We Optimize the Sieve of Eratosthenes for Efficient Prime Number Generation in Python?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










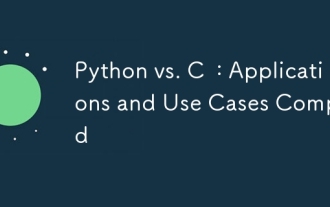
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
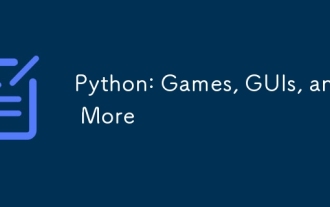
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
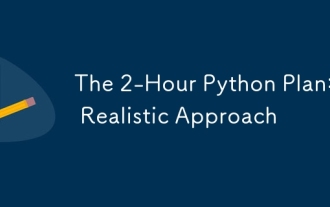
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
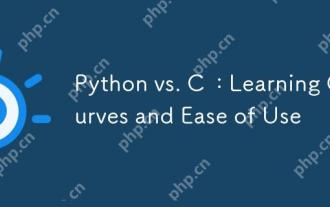
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
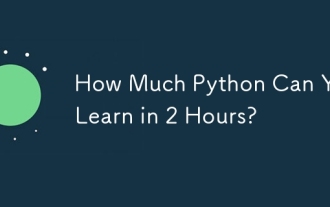
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
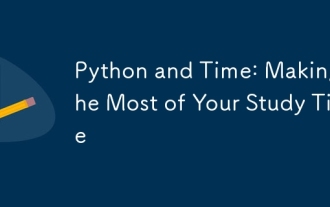
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
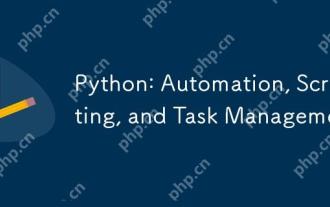
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
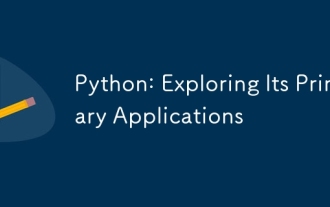
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
