


How can I sort a HashMap by its values while preserving key-value pairs in Java?
Sorting Hashmap by Values
Problem:
We need to sort a HashMap based on the values it contains, and maintain the key-value pairings during sorting.
Solution:
Sorting a HashMap by values can be accomplished using a generic approach. The following steps outline the process:
- Create a Linked List: Convert the HashMap entries into a LinkedList, ensuring that the insertion order is preserved.
- Custom Comparator: Define a custom comparator to compare the values of the entries. It should consider both ascending and descending order options.
- Sort the List: Use the custom comparator to sort the LinkedList based on the values. Since the entries are linked, the keys will also be sorted.
- Convert to Sorted HashMap: Use the sorted LinkedList to construct a new LinkedHashMap, where the keys and values are associated as they were in the original HashMap.
- Custom Version: A tailored version of the sorting method can be created, allowing for specific ascending or descending value ordering.
Example Implementation:
The following Java code implements the sorting algorithm:
import java.util.Collections; import java.util.Comparator; import java.util.HashMap; import java.util.LinkedHashMap; import java.util.LinkedList; import java.util.List; import java.util.Map; import java.util.Map.Entry; public class SortMapByValue { public static final boolean ASC = true; public static final boolean DESC = false; public static void main(String[] args) { // Create dummy HashMap Map<Integer, String> unsortedMap = new HashMap<>(); unsortedMap.put(1, "froyo"); unsortedMap.put(2, "abby"); unsortedMap.put(3, "denver"); unsortedMap.put(4, "frost"); unsortedMap.put(5, "daisy"); // Sort in ascending order Map<Integer, String> sortedMapAsc = sortByValue(unsortedMap, ASC); // Sort in descending order Map<Integer, String> sortedMapDesc = sortByValue(unsortedMap, DESC); // Print sorted maps System.out.println("Sorted Ascending:"); printMap(sortedMapAsc); System.out.println("Sorted Descending:"); printMap(sortedMapDesc); } private static Map<Integer, String> sortByValue(Map<Integer, String> map, boolean order) { List<Entry<Integer, String>> list = new LinkedList<>(map.entrySet()); // Custom comparator for values Collections.sort(list, new Comparator<Entry<Integer, String>>() { public int compare(Entry<Integer, String> o1, Entry<Integer, String> o2) { if (order) { return o1.getValue().compareTo(o2.getValue()); } else { return o2.getValue().compareTo(o1.getValue()); } } }); // Return sorted LinkedHashMap Map<Integer, String> sortedMap = new LinkedHashMap<>(); for (Entry<Integer, String> entry : list) { sortedMap.put(entry.getKey(), entry.getValue()); } return sortedMap; } public static void printMap(Map<Integer, String> map) { for (Entry<Integer, String> entry : map.entrySet()) { System.out.println(entry.getKey() + " - " + entry.getValue()); } System.out.println(); } }
The above is the detailed content of How can I sort a HashMap by its values while preserving key-value pairs in Java?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










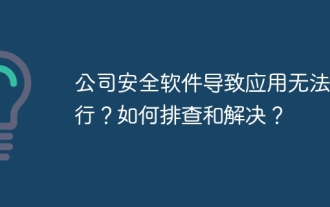
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
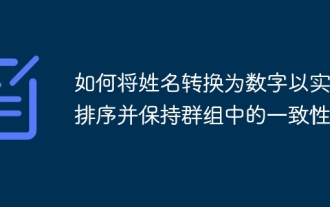
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
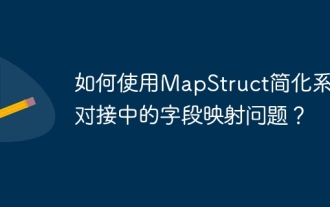
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
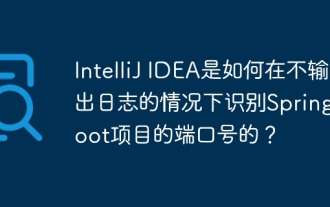
Start Spring using IntelliJIDEAUltimate version...
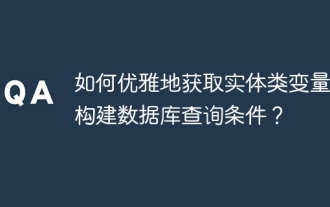
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
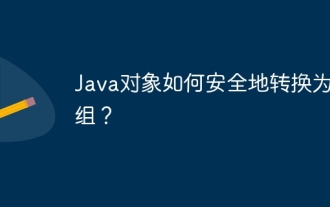
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
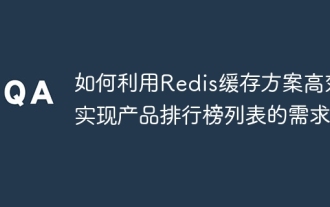
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
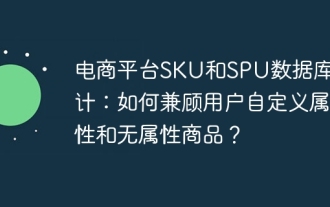
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
