How to Access Members of a JSONArray in Java?
Accessing Members of Items in a JSONArray with Java
When working with JSON data, it's often necessary to access specific values within JSON arrays. A JSONArray represents a list of JSON objects in a JSON document. This article will guide you on how to access and retrieve string values from within a JSONArray using Java.
Consider the following sample JSON:
{ "locations": { "record": [ { "id": 8817, "loc": "NEW YORK CITY" }, { "id": 2873, "loc": "UNITED STATES" }, { "id": 1501 "loc": "NEW YORK STATE" } ] } }
Once you have access to the record JSONArray, you can use the following steps to retrieve the "id" and "loc" values for each record:
- Iterate through the JSONArray: Use the JSONArray.length() method to determine the number of records in the array and use a for loop to iterate through each record.
- Get the JSONObject for each record: For each record in the loop, use the JSONArray.getJSONObject(int) method to retrieve the corresponding JSONObject.
- Extract the values: Use the JSONObject methods to extract the specific values you need. For example, JSONObject.getInt("id") will give you the integer value for the "id" field, and JSONObject.getString("loc") will give you the string value for the "loc" field.
Here's a sample code that demonstrates accessing the "id" and "loc" values:
import org.json.JSONArray; import org.json.JSONObject; ... // Load JSON data as before JSONArray recs = locs.getJSONArray("record"); for (int i = 0; i < recs.length(); ++i) { JSONObject rec = recs.getJSONObject(i); int id = rec.getInt("id"); String loc = rec.getString("loc"); // Process id and loc as needed... }
By following these steps, you can efficiently navigate and extract specific values from within a JSONArray, enabling you to consume and manipulate JSON data in your Java applications.
The above is the detailed content of How to Access Members of a JSONArray in Java?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










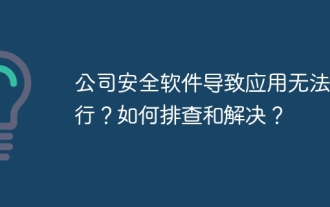
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
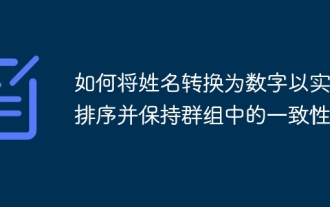
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
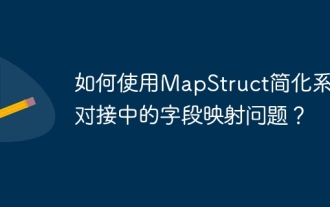
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
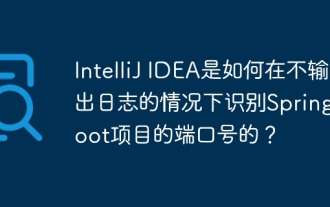
Start Spring using IntelliJIDEAUltimate version...
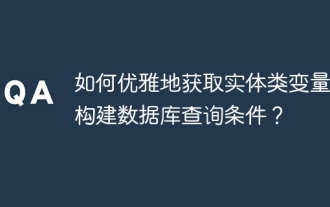
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
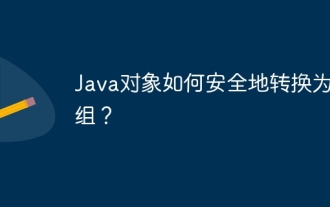
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
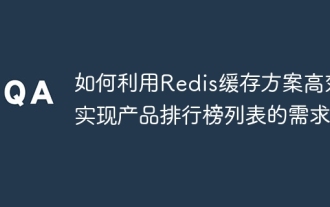
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
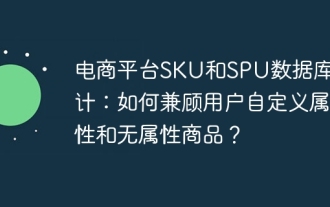
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
