How to Prevent 'No Reachable Servers' Panic in Golang with MGO?
Panic Recovery in Golang with MGO: Preventing "No Reachable Servers" Panic
When connecting to a MongoDB instance using the MGO library in Go, it's possible to encounter a "no reachable servers" panic if the instance is unavailable or offline. This can lead to unexpected program termination.
To prevent this issue, we can implement a recovery mechanism using the defer and recover functions in Go. However, the provided solution in the question is not working effectively.
Modified Code:
Below is a modified version of the given code that successfully recovers from the panic and allows the program to continue execution:
package main import ( "fmt" "time" ) import ( "labix.org/v2/mgo" ) func connectToMongo() bool { fmt.Println("enter main - connecting to mongo") defer func() { if r := recover(); r != nil { fmt.Println("Unable to connect to MongoDB. Received panic:", r) } }() maxWait := time.Duration(5 * time.Second) session, err := mgo.DialWithTimeout("localhost:27017", maxWait) if err != nil { return false } session.SetMode(mgo.Monotonic, true) coll := session.DB("MyDB").C("MyCollection") if coll != nil { fmt.Println("Got a collection object") return true } fmt.Println("Unable to retrieve collection") return false } func main() { if connectToMongo() { fmt.Println("Connected") } else { fmt.Println("Not Connected") } }
In this code, we use a defer function to catch the panic caused by the DialWithTimeout call. If a panic occurs, we print an error message and continue the program's execution, preventing it from terminating prematurely.
Output with MongoDB Down:
When MongoDB is down, the program produces the following output:
enter main - connecting to mongo Unable to connect to MongoDB. Received panic: no reachable servers Not Connected
Output with MongoDB Up:
When MongoDB is up, the program produces the following output:
enter main - connecting to mongo Got a collection object Connected
By catching the panic and providing an informative error message, we can ensure that the program continues to run and can gracefully handle temporary network issues or MongoDB outages.
The above is the detailed content of How to Prevent 'No Reachable Servers' Panic in Golang with MGO?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
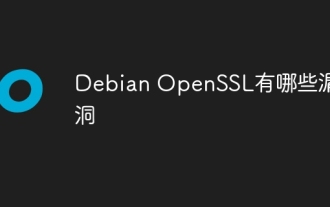
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
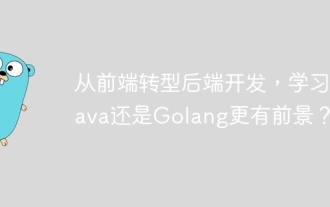
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
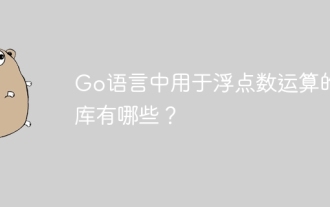
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
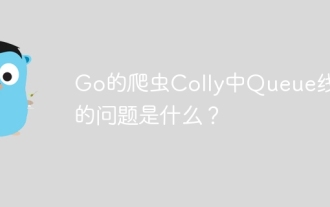
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
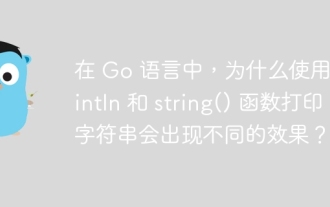
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
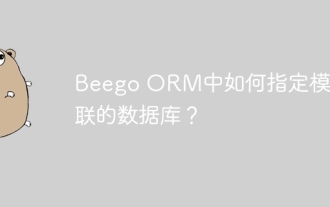
Under the BeegoORM framework, how to specify the database associated with the model? Many Beego projects require multiple databases to be operated simultaneously. When using Beego...
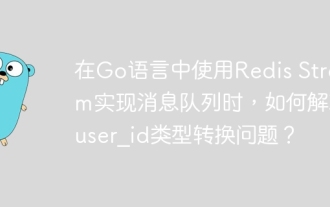
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
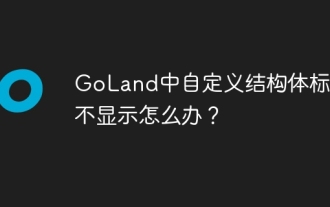
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
