


How Do Method Expressions in Go Facilitate Dynamic Behavior and Code Adaptability?
Method Expressions in Go: Barking Up the Right Tree
In the realm of programming, we often encounter the concept of method expressions. Let's delve into their nature and explore a practical implementation in Go.
Understanding Method Expressions
Method expressions are a concise way to represent a method call as a function. Unlike regular function calls, they require an additional argument, which is the object on which the method will be invoked.
Consider the following Go code:
func main() { dog := Dog{} b := (*Dog).Bark // method expression b(&dog, 5) }
Here, we have a Dog struct with a Bark method. The b variable is assigned a method expression, which effectively binds the Bark method to a function. This allows us to subsequently invoke the method using b.
When to Use Method Expressions
Method expressions provide several advantages:
- Extracting Behavior: They allow us to extract a specific method from an object, making it easier to pass around and handle as a separate unit of behavior.
- Dynamic Binding: In certain scenarios, method expressions can facilitate dynamic binding, where the object that the method is invoked on can vary.
- Adaptability: They enable us to create generic code that can work with different types of objects that share a common method.
Pointer vs. Value Receivers
In the provided code, the Bark method has a pointer receiver (*Dog). This means that the method modifies the dog object itself rather than a copy. If the receiver had been a value receiver (Dog), any changes made to the object inside the method would not be reflected in the original object.
Example: Dynamic Behavior
The following code demonstrates how method expressions can be used to dynamically choose between different behaviors:
func main() { var b func(*Dog, int) if shouldBark { b = (*Dog).Bark } else { b = (*Dog).Sit } d := Dog{} DoAction(b, &d, 3) } func DoAction(f func(*Dog, int), d *Dog, n int) { f(d, n) }
Here, the DoAction function accepts a method expression as an argument and subsequently invokes it on the provided object. This flexibility allows us to dynamically switch between the Bark and Sit methods depending on runtime conditions.
While method expressions can be a powerful tool, it's important to note that they are not widely used in everyday Go programming. They are more suited for advanced scenarios or specialized use cases.
The above is the detailed content of How Do Method Expressions in Go Facilitate Dynamic Behavior and Code Adaptability?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










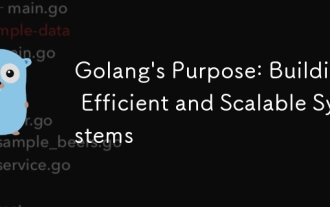
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
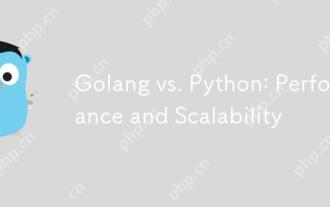
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
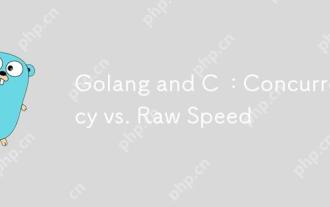
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
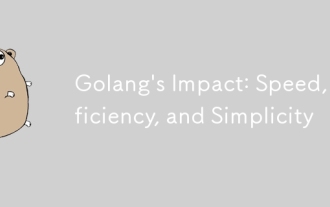
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
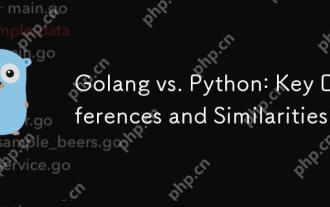
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
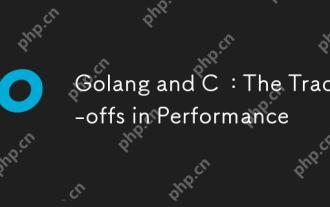
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
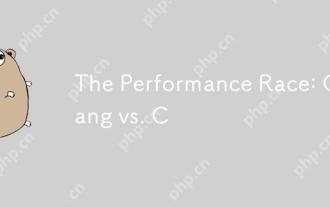
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
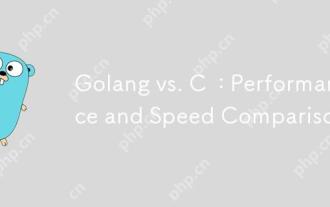
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
