How Can I Use cURL Functionality in Java?
Using cURL in Java
Java does not have built-in cURL capabilities. To use cURL in Java, you must install and integrate a third-party library.
Installation
To install a cURL library for Java, you can choose one of the following options:
- Apache HttpClient: A widely used and comprehensive HTTP library that includes cURL support.
- JCurl: A lightweight and pure Java cURL port.
- HttpComponents: Apache Foundation's modular HTTP library that includes a cURL module.
You can use a package manager such as Maven or Gradle to include the desired library in your project. For example, the Maven dependency for Apache HttpClient is:
<dependency> <groupId>org.apache.httpcomponents</groupId> <artifactId>httpclient</artifactId> <version>4.5.13</version> </dependency>
Usage
Once installed, you can use cURL in Java through the provided classes and methods. Here's an example using Apache HttpClient:
import org.apache.http.HttpEntity; import org.apache.http.client.methods.CloseableHttpResponse; import org.apache.http.client.methods.HttpGet; import org.apache.http.impl.client.CloseableHttpClient; import org.apache.http.impl.client.HttpClientBuilder; public class Main { public static void main(String[] args) throws Exception { CloseableHttpClient client = HttpClientBuilder.create().build(); HttpGet request = new HttpGet("https://stackoverflow.com"); CloseableHttpResponse response = client.execute(request); HttpEntity entity = response.getEntity(); if (entity != null) { // Process the response body } response.close(); client.close(); } }
This code sends an HTTP GET request using cURL to retrieve a web page's contents. You can further explore the documentation of the chosen cURL library for more advanced features and usage scenarios.
The above is the detailed content of How Can I Use cURL Functionality in Java?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










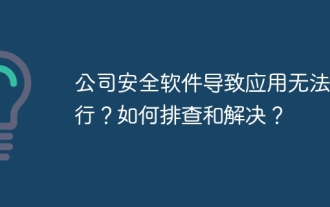
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
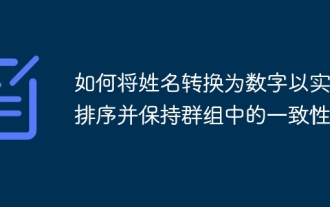
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
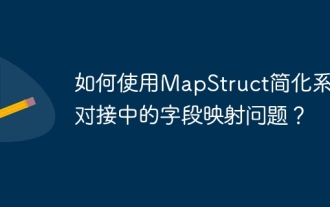
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
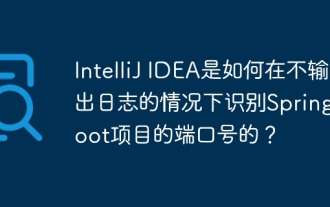
Start Spring using IntelliJIDEAUltimate version...
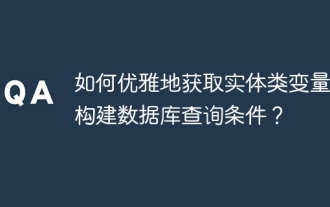
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
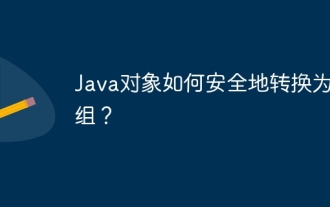
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
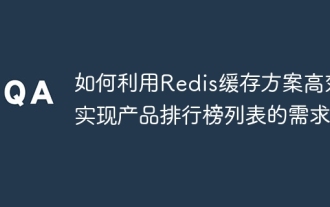
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
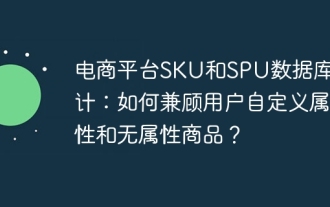
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
