


How to Extract Nested JSON Data from a String Within a Larger JSON Structure?
Accessing Nested Data in Complex JSON with JSON Strings
When working with complex JSON data, you may encounter scenarios where one of the values is another JSON string. This can pose a challenge in extracting the desired data. In this case, you are presented with JSON data that contains an announcement key holding additional JSON data as a string.
To access the "content" field within this nested JSON data, the correct approach is to use the following steps:
import json # Load the raw JSON data raw_replay_data = json.loads('...') # Navigate to the announcement data announcement = raw_replay_data['data']['video_info'][0]['announcement'] # Parse the announcement string as JSON announcement_data = json.loads(announcement) # Extract the desired content content = announcement_data['content'] print(content) # Output: 'FOLLOW ME PLEASE'
Understanding the Data Structure
To grasp the underlying data structure, it is essential to visualize the JSON data in a structured format. Utilizing a tool such as JSONLint or the following code can enhance this understanding:
print(json.dumps(raw_replay_data, indent=4))
Navigating the Ladder of Keys
To effectively access the nested data, you must trace the path through the keys like a ladder:
- data: A dictionary
- video_info: A list of dictionaries
- announcement: A string representing JSON data
- content: The desired field within the parsed JSON data
Loading and Parsing the Nested JSON
Once you have extracted the announcement string, you need to convert it back into Python's JSON data structure. This is achieved by loading the string using the json.loads() function.
Respecting the Data Structure
By following the proper steps outlined above, you ensure that you navigate the data structure correctly. This prevents errors resulting from improper indexing or type conversions.
The above is the detailed content of How to Extract Nested JSON Data from a String Within a Larger JSON Structure?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










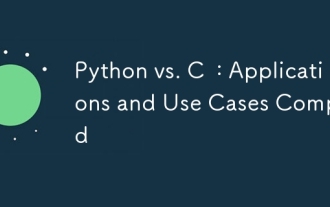
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
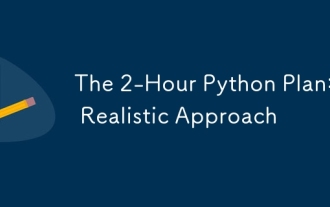
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
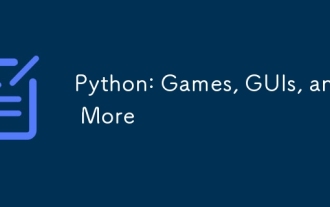
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
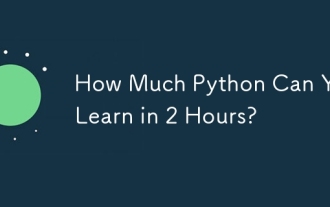
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
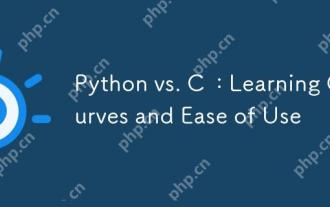
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
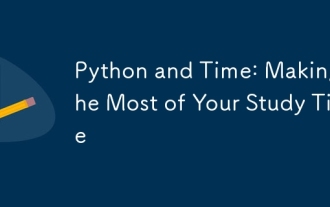
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
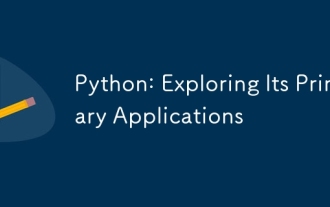
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
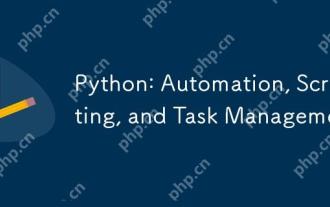
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
