


When Do Parentheses Matter in Python Function and Method Calls?
Unraveling Parenthesis Omission in Function and Method Calls
In Python, functions and methods are treated as first-class objects. This means that they can be assigned to variables, passed as arguments to other functions, and even returned from functions.
However, when we call a function or method, we typically append parentheses to its name, such as my_func(). However, there are certain scenarios where omitting the parentheses can be beneficial.
Consider the following code:
class objectTest(): def __init__(self, a): self.value = a def get_value(self): return self.value a = objectTest(1) b = objectTest(1) print(a == b) print(a.get_value() == b.get_value) print(a.get_value() == b.get_value()) print(a.get_value == b.get_value)
The output of this code is:
False False True False
This puzzling result stems from the fact that get_value is a method, yet we use it like a variable without calling it first. This is possible because omitting the parentheses around a function or method name returns the function or method object itself, known as a callable.
A callable is an object that can be called to execute a specific action when parentheses are added. In the given example, a.get_value refers to a callable object representing the get_value method of the object a.
Therefore, the following comparisons are being made:
- a == b: Compares the object identities of a and b (False)
- a.get_value() == b.get_value: Compares the values returned by calling the get_value method of a and b (False)
- a.get_value() == b.get_value(): Compares the values returned by calling the get_value method of a and b (True)
- a.get_value == b.get_value: Compares the callable objects representing the get_value methods of a and b (False)
Omitting parentheses provides us with flexibility in various scenarios:
- Passing references: When passing callables to other functions or processes, omitting parentheses ensures that the callable is passed as a reference.
- Dynamic calling: In certain contexts, such as using map(), we need to specify a callable and let it be called dynamically.
- Callable collections: We can collect callables in a collection and retrieve them dynamically based on specific criteria.
By understanding the behavior of parentheses omission in function and method calls, we expand our possibilities in Python programming.
The above is the detailed content of When Do Parentheses Matter in Python Function and Method Calls?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










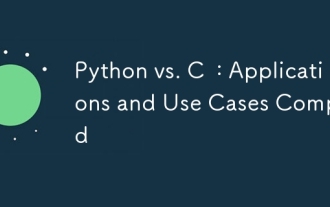
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
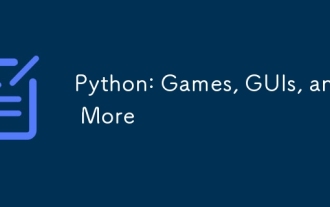
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
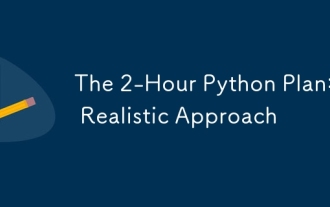
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
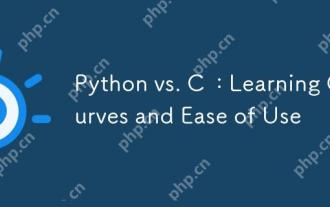
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
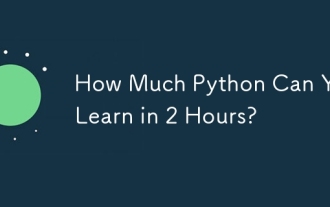
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
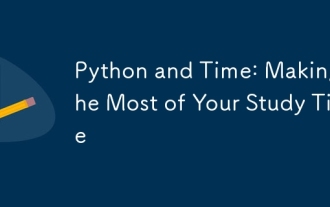
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
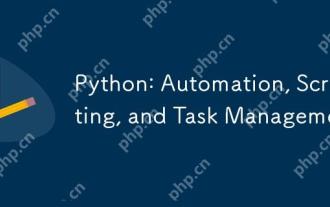
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
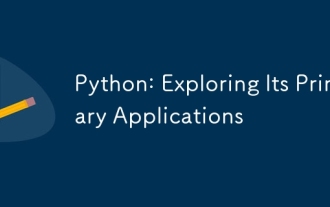
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
