


Can Subclasses Throw Broader Checked Exceptions When Overriding Methods in Java?
Exception Propagation Limitations in Method Overriding
In Java, method overriding grants subclasses the flexibility to implement a different behavior for a method inherited from a superclass. However, this process is governed by certain restrictions, one of which concerns the exception handling capabilities of overriding methods.
Specifically, overriding methods are prohibited from declaring checked exceptions that are broader than those declared by the overridden method. This means that if a superclass method throws a specific checked exception, a subclass implementation cannot declare an exception that is not a subclass of the superclass's exception.
Rationale Behind the Restriction
This restriction exists for reasons of polymorphism and type safety. When a subclass overrides a method and changes the exception behavior, it could unintentionally break the contract established by the superclass.
Consider the following example:
class Superclass { public void doSomething() throws IOException { // Implementation } } class Subclass extends Superclass { @Override public void doSomething() throws SQLException { // Not allowed // Implementation } }
If we allowed the doSomething() method in Subclass to throw a broader exception (SQLException), it could potentially undermine the assumption made by code that calls the doSomething() method through a reference to the superclass.
In the above scenario, a try-catch block that handles IOException in the calling code would not catch the SQLException thrown by the overriding method. This could lead to unexpected errors and program crashes.
Implications for Exception Handling
The restriction on broader exceptions in overriding methods ensures that subclasses can only expand the set of potential exceptions thrown by the overridden method, not introduce new or broader exceptions.
Additionally, unchecked exceptions (such as NullPointerException or IllegalArgumentException) are not subject to this rule. Subclass methods are free to declare unchecked exceptions regardless of whether the overridden method declares them.
The above is the detailed content of Can Subclasses Throw Broader Checked Exceptions When Overriding Methods in Java?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










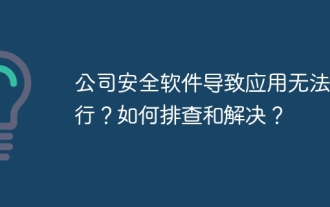
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
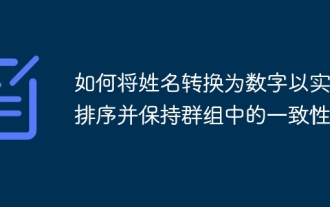
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
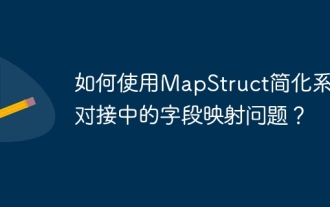
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
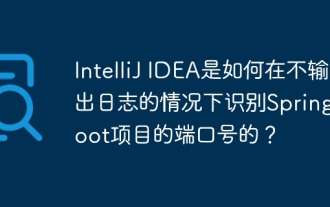
Start Spring using IntelliJIDEAUltimate version...
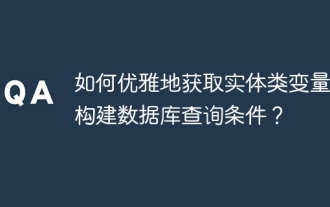
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
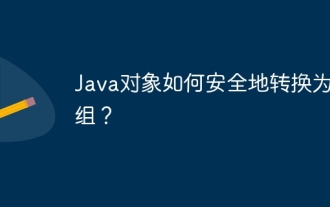
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
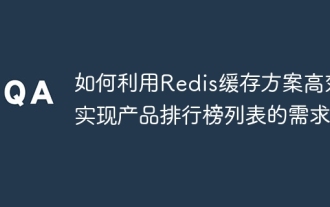
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
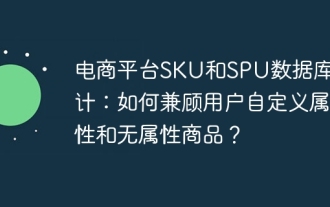
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
