


How to Ensure All Goroutines Complete When Using a Buffered Channel as a Semaphore?
Waiting for an Empty Buffered Channel (Semaphore)
In concurrent programming, it's crucial to control how many goroutines execute simultaneously. One approach is to use a buffered channel as a semaphore. However, a common issue arises when waiting for the semaphore to drain completely.
Problem:
Let's say we have a slice of integers that we want to process concurrently, with a limit of two concurrent go routines. We use a buffered channel as a semaphore to enforce this limit. While the code works for most integers, the program exits prematurely before the last go routines finish due to the buffer never reaching emptiness.
The Question:
How do we wait for the buffered channel to drain completely, ensuring all go routines complete before the program exits?
Answer:
Using a channel to wait for a specific condition is not feasible due to the lack of a race-free way to check its length. Instead, we can employ a sync.WaitGroup to monitor the completion of all goroutines.
Resolved Code:
sem := make(chan struct{}, 2) var wg sync.WaitGroup for _, i := range ints { wg.Add(1) // acquire semaphore sem <- struct{}{} // start long running go routine go func(id int) { defer wg.Done() // do something // release semaphore <-sem }(i) } wg.Wait()
By using sync.WaitGroup, we can wait for all goroutines to finish before the program exits, ensuring that all processing is complete.
The above is the detailed content of How to Ensure All Goroutines Complete When Using a Buffered Channel as a Semaphore?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
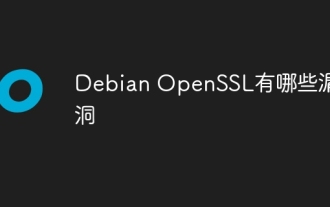
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
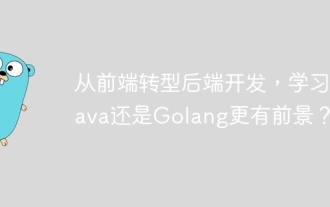
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
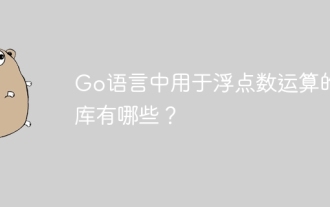
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
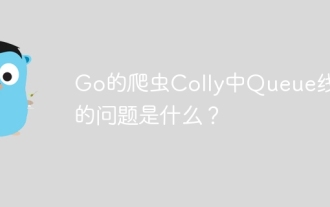
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
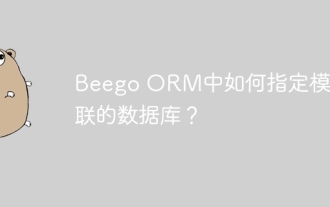
Under the BeegoORM framework, how to specify the database associated with the model? Many Beego projects require multiple databases to be operated simultaneously. When using Beego...
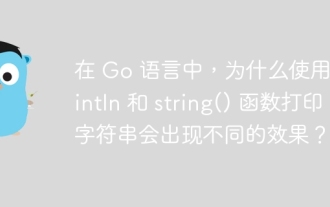
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
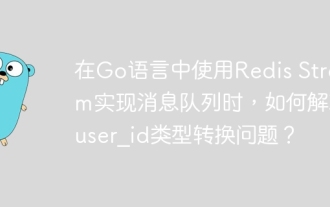
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
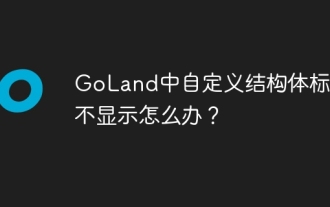
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
