How to Efficiently Import Modules from a Python Folder?
Importing Modules from a Folder
When organizing your Python code, it often becomes necessary to import multiple modules from a specific folder. One common approach involves converting the folder into a Python package by adding an __init__.py file at the root.
The __init__.py Approach
By creating an __init__.py file, you can import the modules within the folder as follows:
from my_package import *
However, this approach may not always be ideal, as it imports all modules from the folder, irrespective of whether you need them or not.
Importing Specific Modules Dynamically
To selectively import modules from a folder, you can utilize the following code:
import os import inspect # Get the current folder path folder_path = os.path.dirname(os.path.abspath(inspect.getfile(inspect.currentframe()))) # Iterate over all .py files in the folder for file_name in os.listdir(folder_path): if file_name.endswith('.py') and file_name != '__init__.py': # Import the module using dynamic import module_name = file_name[:-3] module = __import__(module_name, fromlist=['*'])
Using this method, you can import specific modules within the folder by accessing them via the module_name variable.
The above is the detailed content of How to Efficiently Import Modules from a Python Folder?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


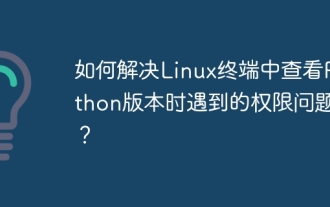
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
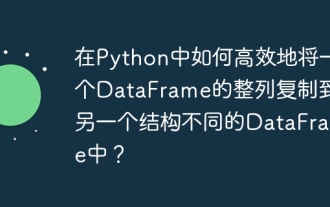
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
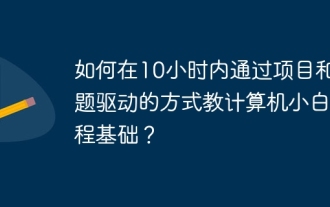
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
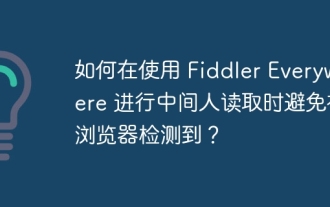
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
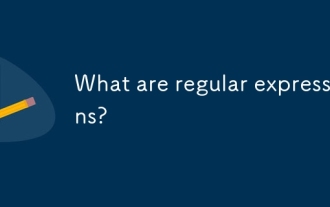
Regular expressions are powerful tools for pattern matching and text manipulation in programming, enhancing efficiency in text processing across various applications.
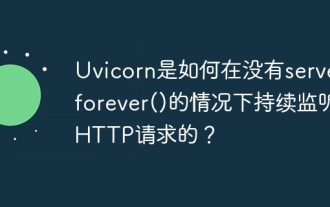
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
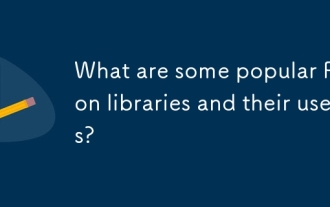
The article discusses popular Python libraries like NumPy, Pandas, Matplotlib, Scikit-learn, TensorFlow, Django, Flask, and Requests, detailing their uses in scientific computing, data analysis, visualization, machine learning, web development, and H
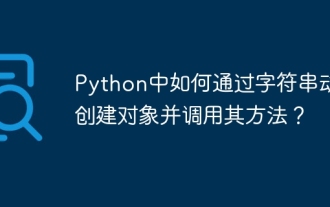
In Python, how to dynamically create an object through a string and call its methods? This is a common programming requirement, especially if it needs to be configured or run...
