Why and When Should You Use `db.Close()` in Go?
Understanding the Necessity of db.Close() in Go
The primary objective of this discussion revolves around the necessity of invoking the db.Close() method in Go. The question arises from a beginner's perspective, highlighting concerns about proper database connection management and potential consequences if db.Close() is omitted.
To address these concerns effectively, it's crucial to clarify that database connections in Go are inherently handled by a connection pool within the sql.DB type. This means that once a connection is established with sql.Open(), the driver creates a pool of idle connections for concurrent utilization by multiple goroutines.
Importance of Connection Pooling
The rationale behind connection pooling is to optimize resource allocation, particularly in high-traffic scenarios. Instead of establishing a new connection for each request, the pool allows efficient reuse of existing connections, minimizing overhead and facilitating scalability.
Automatic Connection Closure
A significant takeaway is that it's not mandatory to explicitly close the database connection using db.Close(). This is because the connection pool automatically manages the lifecycle of connections. When the program exits, all active connections in the pool are gracefully closed, ensuring proper resource cleanup.
Graceful Shutdown for Abnormal Termination
While automatic connection closure is generally sufficient, there may be edge cases where you desire explicit control over database connection shutdown. For these scenarios, you can define a custom CloseDB() function to manually invoke db.Close(), ensuring that all connections are closed, even in the event of an abnormal program termination.
Code Example for Graceful Shutdown
func CloseDB() error { return db.Close() } func main() { // ... (application setup and execution) ... if err := CloseDB(); err != nil { // Handle error accordingly } }
Conclusion
In conclusion, while invoking db.Close() is not inherently required in Go, it provides an additional level of control for scenarios involving abnormal program termination. However, for typical applications, the connection pool efficiently manages database connections, ensuring proper cleanup upon program exit.
The above is the detailed content of Why and When Should You Use `db.Close()` in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










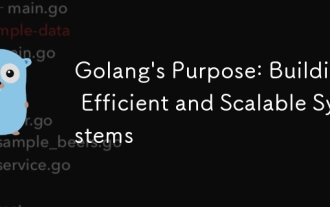
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
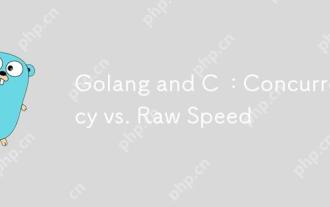
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
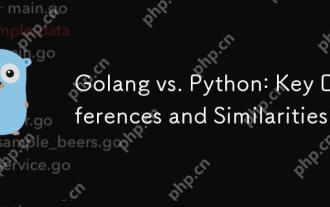
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
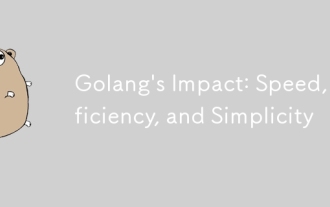
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
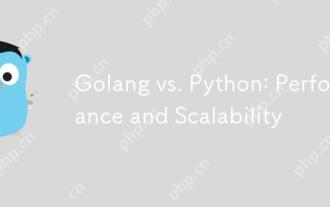
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
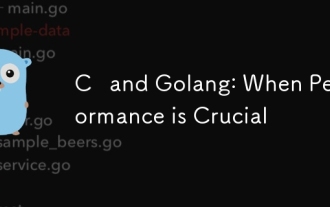
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
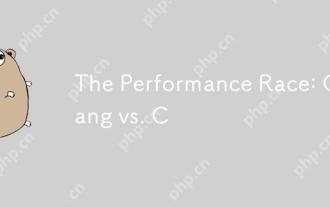
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
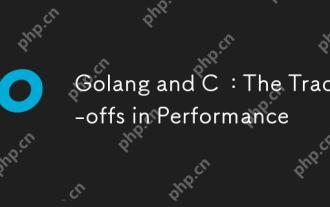
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
