


How Can I Use Reflection to Call the Variadic `Rows.Scan()` Function in Go?
Calling the Variadic Scan Function Using Reflection
To call the Rows.Scan() function using reflection, you can leverage the power of reflection to pass a variable number of pointers to the function. This is particularly useful when you want to fill a slice with values from a database query result.
Steps to Implement
- Retrieve the column names from the query result using rows.Columns(). This will give you the length of the row.
- Create a slice of []interface{} to store the data points, and another slice of pointers to store the addresses of these data points.
- Call the reflect.ValueOf method on the slice of pointers to obtain a reflect.Value object.
- Use the Addr method to obtain a slice of values that represent the addresses of the data points.
- Call rows.Scan and pass it the slice of addresses as arguments.
- After calling Scan, the slice of []interface{} will be filled with the data points.
Sample Code
package main import ( "fmt" "reflect" "database/sql" ) func main() { // Open a database connection. db, _ := sql.Open("postgres", "user=postgres dbname=database password=password") // Query the database. rows, _ := db.Query("SELECT * FROM table_name") // Get the column names. columns, _ := rows.Columns() columnCount := len(columns) // Create slices to store data points and pointers. data := make([]interface{}, columnCount) dataPtrs := make([]interface{}, columnCount) // Obtain a slice of pointers. pointers := reflect.ValueOf(dataPtrs) // Obtain a slice of addresses. addresses := pointers.Addr() // Fill the data points by calling Rows.Scan(). rows.Scan(addresses...) // Print the data points. for i := 0; i < columnCount; i++ { fmt.Println(columns[i], data[i]) } }
This code snippet demonstrates how to use reflection to call the Rows.Scan() function with a variable number of pointers. It dynamically retrieves the column names from the query result and creates slices to store the data points and pointers. By using reflection to obtain the slice of addresses, you can pass it to rows.Scan and fill the data points accordingly.
The above is the detailed content of How Can I Use Reflection to Call the Variadic `Rows.Scan()` Function in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










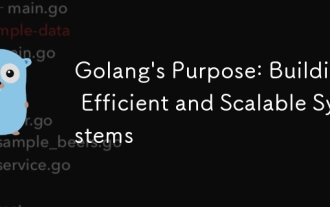
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
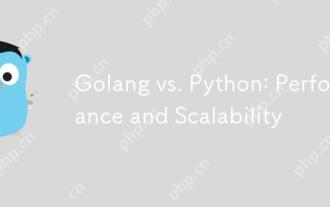
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
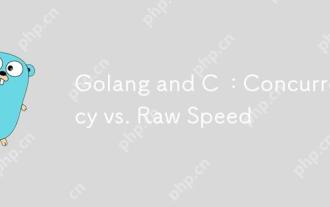
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
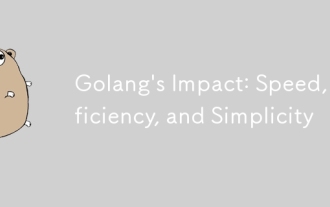
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
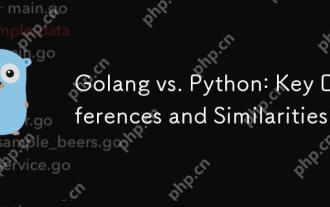
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
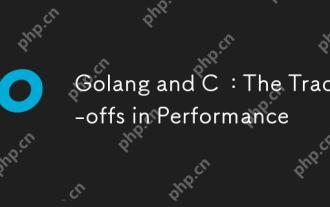
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
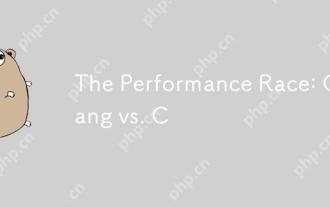
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
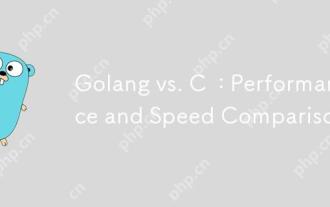
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
