


Why Do Python Lexical Closures in Loops Produce Unexpected Results?
Lexical Closures in Python: Understanding the Unexpected Behavior
In Python, lexical closures allow inner functions to access variables defined in the enclosing scope. However, an unexpected behavior arises when defining functions within a loop that modifies a shared variable. To illustrate this, consider the following Python code:
flist = [] for i in range(3): def func(x): return x * i flist.append(func) for f in flist: print(f(2))
This code prints "4 4 4," which is surprising as one might expect "0 2 4." The reason for this unexpected behavior lies in the closure mechanism. In Python, functions defined within loops create new functions but share the enclosing scope, which in this case is the global scope. As a result, when the value of i is subsequently modified, all the functions within the list refer to the same modified i value.
To address this issue, one needs to create unique environments for each function within the loop. This can be accomplished by using a function creater:
flist = [] for i in range(3): def funcC(j): def func(x): return x * j return func flist.append(funcC(i)) for f in flist: print(f(2))
In this revised code, each call to funcC generates a new closure environment with its own value of i. As a result, each function in the list has access to a distinct i value, leading to the expected output of "0 2 4."
This behavior underscores the importance of understanding how closures work in Python, especially when dealing with side effects and functional programming. When functions defined within loops share a modified variable, unexpected behavior can arise. Utilizing function creators helps create unique environments for each function and ensures the desired behavior is achieved.
The above is the detailed content of Why Do Python Lexical Closures in Loops Produce Unexpected Results?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










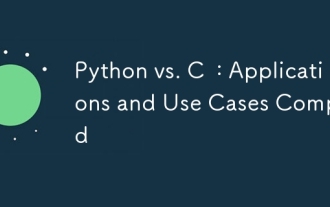
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
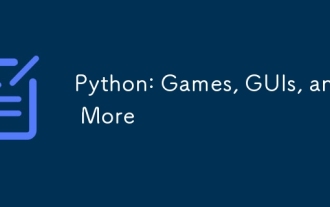
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
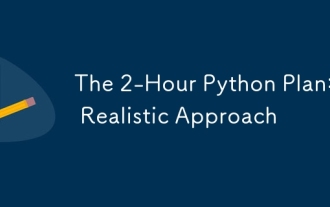
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
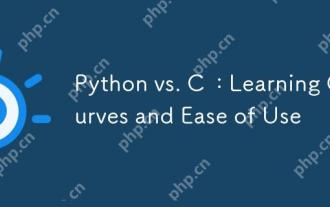
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
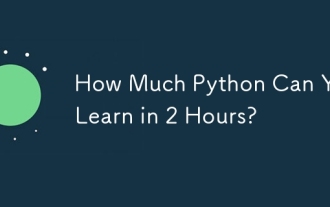
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
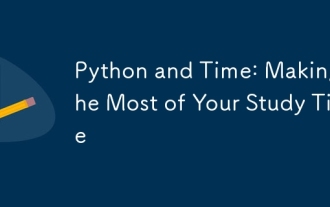
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
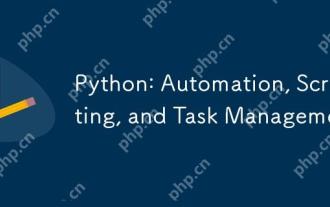
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
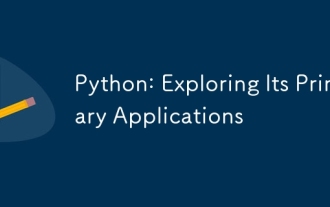
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
