


How Can I Implement an Interface with Unexported Methods in a Different Go Package?
Implementing Interfaces with Unexported Methods Across Packages
You are attempting to restrict access to an interface's implementation details by making its methods unexported. While this is a valid approach, it can pose challenges when implementing the interface in a separate package.
The Issue
The compiler raises an error because it cannot access the unexported getInvoice method of accountingsystem.Adapter. This is due to the Go language's visibility rules, which prevent packages from using unexported identifiers outside their own scope.
Possible Solution
Unfortunately, there is no direct way to implement an interface with unexported methods in another package. However, there are alternative methods to achieve similar results.
Using Anonymous Struct Fields
You can embed the interface as an anonymous field within a struct in the separate package. While this allows the struct to satisfy the interface, it also makes it impossible to define your own implementation of the unexported methods.
Registering the Adapter
A more suitable approach is to make the adapter type unexported and provide a function within the adapter package that registers it with the main package. This allows you to control access to the adapter while still maintaining flexibility.
// accountingsystem package type adapter struct {} // ... implementation omitted ... func SetupAdapter() { accounting.SetAdapter(adapter{}) } // main package func main() { accountingsystem.SetupAdapter() }
By using this method, you can restrict access to the adapter's implementation while ensuring that the interface can be used across packages.
The above is the detailed content of How Can I Implement an Interface with Unexported Methods in a Different Go Package?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










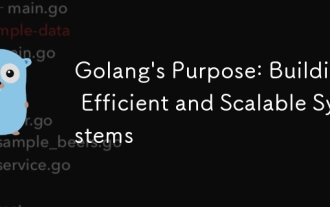
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
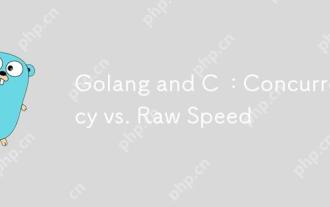
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
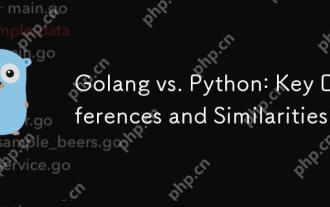
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
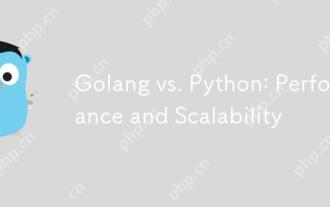
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
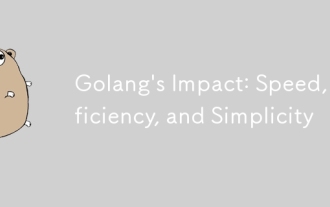
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
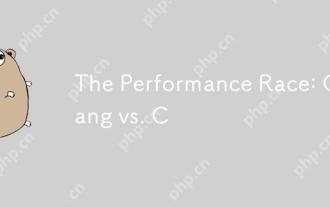
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
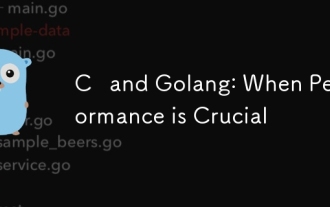
C is more suitable for scenarios where direct control of hardware resources and high performance optimization is required, while Golang is more suitable for scenarios where rapid development and high concurrency processing are required. 1.C's advantage lies in its close to hardware characteristics and high optimization capabilities, which are suitable for high-performance needs such as game development. 2.Golang's advantage lies in its concise syntax and natural concurrency support, which is suitable for high concurrency service development.
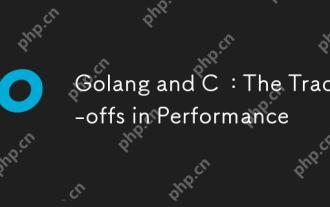
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
