How to Avoid ConcurrentModificationException When Adding to an ArrayList?
Concurrent Modification Exception: Adding to an ArrayList
The ConcurrentModificationException is raised when you attempt to modify a collection while iterating over it. This error occurs when you modify the collection by adding or removing elements while traversing it using an Iterator.
Cause of the Exception
In the provided code snippet:
for (Iterator<Element> it = mElements.iterator(); it.hasNext();){ Element element = it.next(); // Code to check and add new elements }
Within the loop, the code attempts to add new elements to the mElements ArrayList while iterating over it using an Iterator. This triggers the ConcurrentModificationException because the collection is modified during iteration.
Solution 1: Use a Temporary List
To resolve this issue, you can use a temporary list to store the new elements that need to be added to the ArrayList. After finishing the iteration, you can add the elements from the temporary list to the ArrayList.
// Create a new list to store any new elements that need to be added List<Element> thingsToBeAdded = new ArrayList<>(); // Iterate over the mElements list for (Iterator<Element> it = mElements.iterator(); it.hasNext();) { Element element = it.next(); // Code to check and mark elements for addition (e.g., set cFlag) if (element.cFlag) { // Add the new element to the temporary list thingsToBeAdded.add(new Element("crack", getResources(), (int) touchX, (int) touchY)); element.cFlag = false; } } // Add the new elements to the mElements list after finishing the iteration mElements.addAll(thingsToBeAdded);
Solution 2: Use an Enhanced For-Each Loop
An alternative approach is to use an enhanced for-each loop, which provides a safer way to iterate over a collection. The enhanced for-each loop uses an indirection abstraction to ensure that the collection is not modified during iteration.
for (Element element : mElements) { // Code to check and modify elements (e.g., set cFlag) }
In this case, you would need to update the code that adds new elements separately after the iteration.
The above is the detailed content of How to Avoid ConcurrentModificationException When Adding to an ArrayList?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


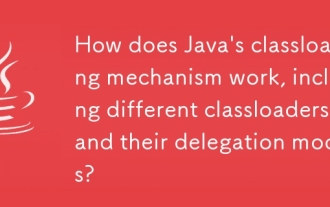
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa
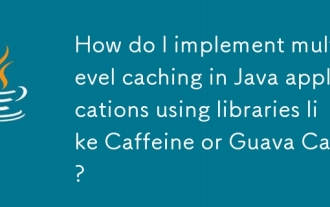
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra
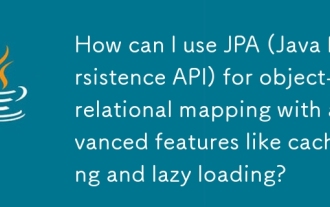
The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
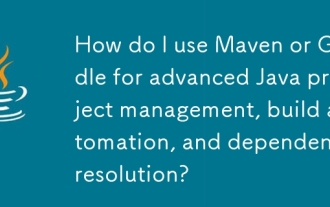
The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
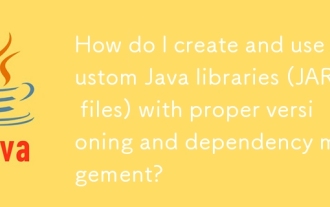
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
