How to Efficiently Create Deep Copies of Maps in Go?
Making Copies of Maps in Go: Is There a Built-In Function?
Maps in Go are a versatile data structure, but the lack of a built-in function for creating copies can be a hurdle. Fortunately, several approaches can help you achieve this.
Using the encoding/gob Package
For a general solution, the encoding/gob package provides a robust way to make deep copies of complex data structures, including maps. By encoding the original map and then decoding it into a new variable, you create a copy that references a different memory location.
package main import ( "bytes" "encoding/gob" "fmt" "log" ) func main() { ori := map[string]int{ "key": 3, "clef": 5, } var mod bytes.Buffer enc := gob.NewEncoder(&mod) dec := gob.NewDecoder(&mod) fmt.Println("ori:", ori) // key:3 clef:5 err := enc.Encode(ori) if err != nil { log.Fatal("encode error:", err) } var cpy map[string]int err = dec.Decode(&cpy) if err != nil { log.Fatal("decode error:", err) } fmt.Println("cpy:", cpy) // key:3 clef:5 cpy["key"] = 2 fmt.Println("cpy:", cpy) // key:2 clef:5 fmt.Println("ori:", ori) // key:3 clef:5 }
By using encoding/gob, you can create deep copies that respect even nested structures, like slices of maps or maps containing slices.
Other Considerations
While encoding/gob offers a general solution, it may not be suitable for all cases. If your maps are relatively simple, you may consider writing a custom function for creating shallow copies. Additionally, there are third-party libraries that provide map copying functionality.
Remember that copies in Go are always deep, meaning they create new instances of the data, unlike shallow copies, which only create references to the original. This is an important distinction to consider when manipulating maps.
The above is the detailed content of How to Efficiently Create Deep Copies of Maps in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


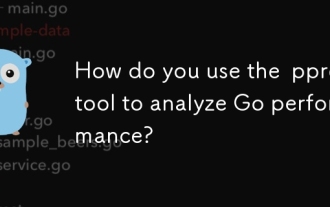
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
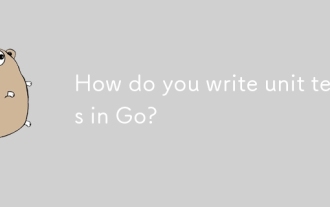
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
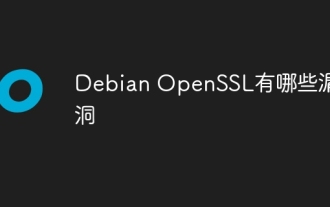
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
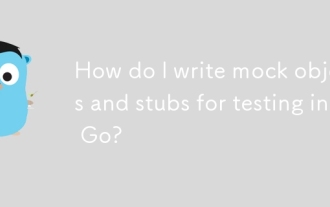
This article demonstrates creating mocks and stubs in Go for unit testing. It emphasizes using interfaces, provides examples of mock implementations, and discusses best practices like keeping mocks focused and using assertion libraries. The articl
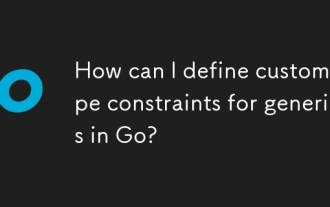
This article explores Go's custom type constraints for generics. It details how interfaces define minimum type requirements for generic functions, improving type safety and code reusability. The article also discusses limitations and best practices
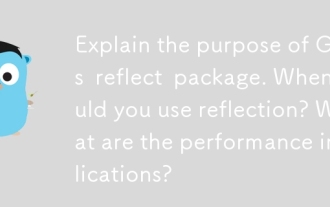
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
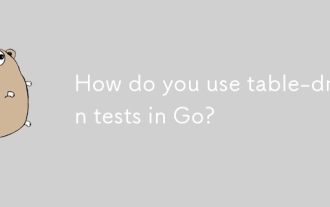
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a
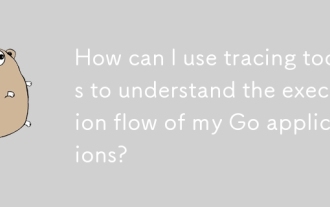
This article explores using tracing tools to analyze Go application execution flow. It discusses manual and automatic instrumentation techniques, comparing tools like Jaeger, Zipkin, and OpenTelemetry, and highlighting effective data visualization
