


How Can I Automate the Deletion of Data Older Than Two Hours in Firebase?
Automating Data Cleanup in Firebase
To efficiently delete Firebase data that is older than two hours, consider the following approach:
Firebase Limitations:
Firebase does not offer queries with dynamic parameters like "two hours ago." However, it can execute queries for specific values, such as "after a particular timestamp."
Time-Based Deletion:
Implement a code snippet that executes periodically to delete data that is older than two hours at that time.
var ref = firebase.database().ref('/path/to/items/'); var now = Date.now(); var cutoff = now - 2 * 60 * 60 * 1000; var old = ref.orderByChild('timestamp').endAt(cutoff).limitToLast(1); var listener = old.on('child_added', function(snapshot) { snapshot.ref.remove(); });
Implementation Details:
- Use child_added instead of value and limitToLast(1) to handle deletions efficiently.
- As each child is deleted, Firebase will trigger child_added for the new "last" item until there are no more items after the cutoff point.
Cloud Functions for Firebase:
If you want this code to run periodically in the background, you can use Cloud Functions for Firebase:
exports.deleteOldItems = functions.database.ref('/path/to/items/{pushId}') .onWrite((change, context) => { var ref = change.after.ref.parent; var now = Date.now(); var cutoff = now - 2 * 60 * 60 * 1000; var oldItemsQuery = ref.orderByChild('timestamp').endAt(cutoff); return oldItemsQuery.once('value', function(snapshot) { var updates = {}; snapshot.forEach(function(child) { updates[child.key] = null; }); return ref.update(updates); }); });
Note:
- This function will trigger whenever data is written to /path/to/items, only deleting child nodes that meet the time criteria.
- The code is also available in the functions-samples repo for your convenience.
The above is the detailed content of How Can I Automate the Deletion of Data Older Than Two Hours in Firebase?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


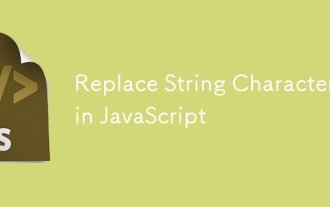
Detailed explanation of JavaScript string replacement method and FAQ This article will explore two ways to replace string characters in JavaScript: internal JavaScript code and internal HTML for web pages. Replace string inside JavaScript code The most direct way is to use the replace() method: str = str.replace("find","replace"); This method replaces only the first match. To replace all matches, use a regular expression and add the global flag g: str = str.replace(/fi
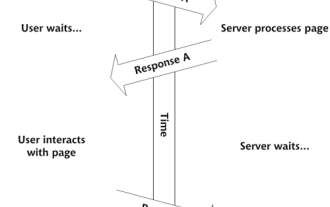
So here you are, ready to learn all about this thing called AJAX. But, what exactly is it? The term AJAX refers to a loose grouping of technologies that are used to create dynamic, interactive web content. The term AJAX, originally coined by Jesse J
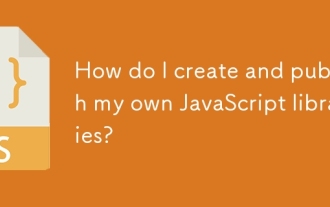
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
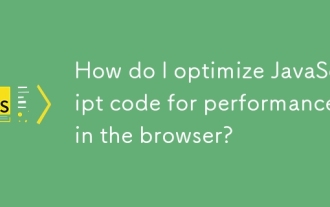
The article discusses strategies for optimizing JavaScript performance in browsers, focusing on reducing execution time and minimizing impact on page load speed.
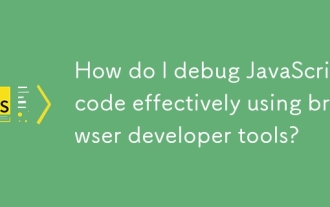
The article discusses effective JavaScript debugging using browser developer tools, focusing on setting breakpoints, using the console, and analyzing performance.
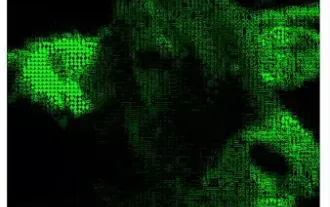
Bring matrix movie effects to your page! This is a cool jQuery plugin based on the famous movie "The Matrix". The plugin simulates the classic green character effects in the movie, and just select a picture and the plugin will convert it into a matrix-style picture filled with numeric characters. Come and try it, it's very interesting! How it works The plugin loads the image onto the canvas and reads the pixel and color values: data = ctx.getImageData(x, y, settings.grainSize, settings.grainSize).data The plugin cleverly reads the rectangular area of the picture and uses jQuery to calculate the average color of each area. Then, use
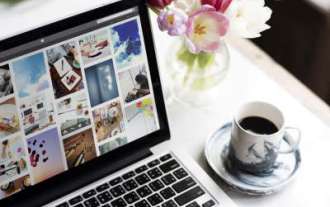
This article will guide you to create a simple picture carousel using the jQuery library. We will use the bxSlider library, which is built on jQuery and provides many configuration options to set up the carousel. Nowadays, picture carousel has become a must-have feature on the website - one picture is better than a thousand words! After deciding to use the picture carousel, the next question is how to create it. First, you need to collect high-quality, high-resolution pictures. Next, you need to create a picture carousel using HTML and some JavaScript code. There are many libraries on the web that can help you create carousels in different ways. We will use the open source bxSlider library. The bxSlider library supports responsive design, so the carousel built with this library can be adapted to any
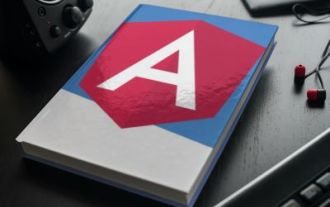
Data sets are extremely essential in building API models and various business processes. This is why importing and exporting CSV is an often-needed functionality.In this tutorial, you will learn how to download and import a CSV file within an Angular
