


How to Sort Strings Containing Numbers Naturally in Python?
Sorting Strings with Embedded Numbers
When dealing with strings that contain numbers, sorting them alphabetically may not yield the desired results. To correctly sort such strings, you need to consider both the textual and numerical components.
Human Sorting (Natural Sorting)
Natural sorting, also known as human sorting, is a technique used to sort items in a way that is consistent with how humans perceive them. This approach involves extracting the numbers from the strings and then sorting them based on their numerical values.
In Python, you can implement natural sorting using the following custom sorting key:
def natural_keys(text): return [int(c) if c.isdigit() else c for c in re.split(r'(\d+)', text)]
This function splits the string into characters and numbers and then converts the numerical characters to integers. The sorted list is obtained by calling the sort() function with this custom key:
alist = ["something1", "something12", "something17", "something2", "something25", "something29"] alist.sort(key=natural_keys) print(alist)
The output would be:
['something1', 'something2', 'something12', 'something17', 'something25', 'something29']
Sorting Strings with Floats
If your strings contain floating-point numbers, you can modify the natural sorting key to extract and convert to floats using the following regex:
def natural_keys(text): return [float(c) if c.isdigit() else c for c in re.split(r'[+-]?([0-9]+(?:[.][0-9]*)?|[.][0-9]+)', text)]
This modified key allows you to sort strings with floating-point numbers as well:
alist = ["something1", "something2", "something1.0", "something1.25", "something1.105"] alist.sort(key=natural_keys) print(alist)
The output would be:
['something1', 'something1.0', 'something1.105', 'something1.25', 'something2']
By utilizing human sorting techniques, you can efficiently sort strings with embedded numbers in a way that aligns with human intuition.
The above is the detailed content of How to Sort Strings Containing Numbers Naturally in Python?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


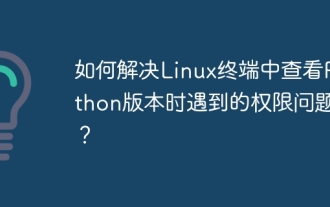
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
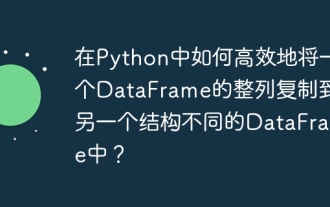
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
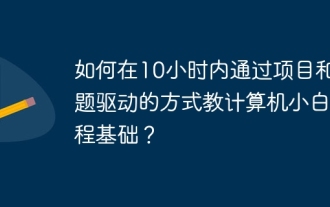
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
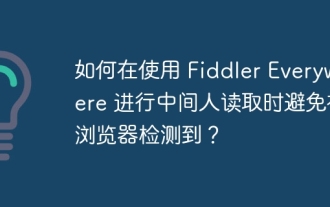
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
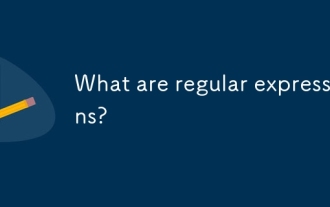
Regular expressions are powerful tools for pattern matching and text manipulation in programming, enhancing efficiency in text processing across various applications.
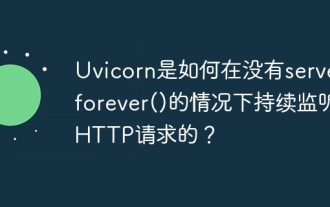
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
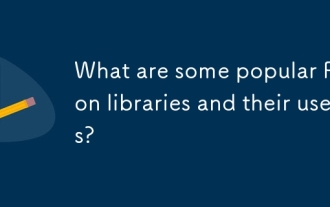
The article discusses popular Python libraries like NumPy, Pandas, Matplotlib, Scikit-learn, TensorFlow, Django, Flask, and Requests, detailing their uses in scientific computing, data analysis, visualization, machine learning, web development, and H
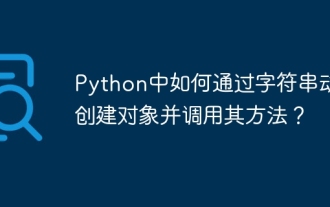
In Python, how to dynamically create an object through a string and call its methods? This is a common programming requirement, especially if it needs to be configured or run...
