How to Properly Implement Hidden Interface Methods in Go?
Implementing Interfaces with Hidden Methods
When developing an accounting system, it can be desirable to hide specific implementations of an interface from the main program to ensure that only one accounting system is active. To achieve this, one may consider making the interface methods unexported and creating exported functions that call a function from a local adapter.
package accounting import "errors" type IAdapter interface { getInvoice() error } var adapter IAdapter func SetAdapter(a IAdapter) { adapter = a } func GetInvoice() error { if (adapter == nil) { return errors.New("No adapter set!") } return adapter.getInvoice() }
However, this approach encounters a compilation error because the compiler cannot access the unexported getInvoice method from the accountingsystem package.
cannot use adapter (type accountingsystem.Adapter) as type accounting.IAdapter in argument to accounting.SetAdapter: accountingsystem.Adapter does not implement accounting.IAdapter (missing accounting.getInvoice method) have accountingsystem.getInvoice() error want accounting.getInvoice() error
Anonymous Struct Field Approach
One possible solution is to use anonymous struct fields. While this allows the accountingsystem.Adapter to satisfy the accounting.IAdapter interface, it prevents the user from providing their own implementation of the unexported method.
type Adapter struct { accounting.IAdapter }
Alternative Approach
A more idiomatic approach is to create an unexported adapter type and provide a function for registering the adapter with the accounting package.
package accounting type IAdapter interface { GetInvoice() error } package accountingsystem type adapter struct {} func (a adapter) GetInvoice() error {return nil} func SetupAdapter() { accounting.SetAdapter(adapter{}) }
By using this approach, the accountingsystem.adapter type is hidden from the main program, and the accounting system can be initialized by calling the SetupAdapter function.
The above is the detailed content of How to Properly Implement Hidden Interface Methods in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
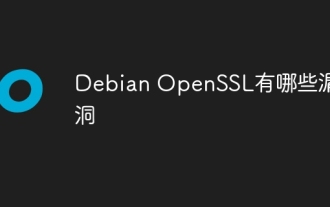
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
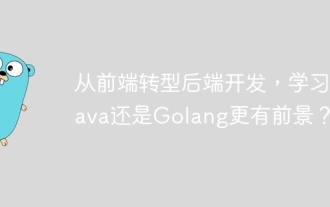
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
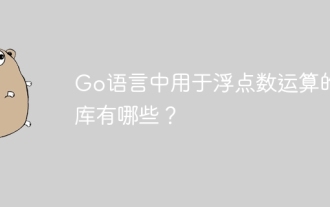
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
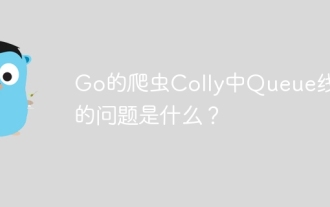
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
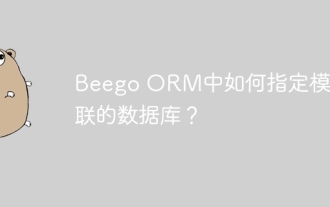
Under the BeegoORM framework, how to specify the database associated with the model? Many Beego projects require multiple databases to be operated simultaneously. When using Beego...
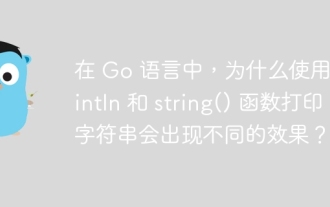
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
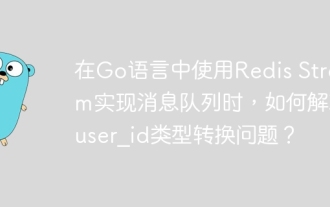
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
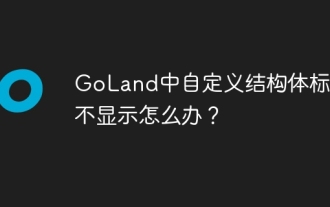
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
