


How to Extract Activations from Specific Layers in a Keras Model?
How to Get Output of Each Layer in Keras
When working with Deep Neural Networks (DNNs), it is often useful to inspect the activations of individual layers. This can help you understand the model's behavior and identify potential issues. Keras, a popular DNN library for Python, provides a simple way to achieve this.
Using the Model Layers Interface
Keras models are constructed as a sequence of layers, each performing a specific operation on the input. To retrieve the output of a particular layer, you can use the following syntax:
model.layers[index].output
where index is the index of the layer you want to extract the output from. For example, to get the output of the second convolutional layer in the provided code snippet:
conv_output = model.layers[2].output
Getting Output from All Layers
To extract the output from all layers in the model, you can use a list comprehension:
outputs = [layer.output for layer in model.layers]
Creating Evaluation Functions
To actually evaluate the outputs of the layers, Keras provides a set of functions called K.function. These functions take as input a list of tensors and return a list of outputs.
To create an evaluation function for each layer output, you can do the following:
from keras import backend as K functors = [K.function([inp, K.learning_phase()], [out]) for out in outputs]
where inp is the input tensor, K.learning_phase() is a flag to indicate whether the model is in training or inference mode, and out is the output of the layer.
Evaluating Layer Outputs
Now, you can evaluate the layer outputs by passing the input data to the corresponding evaluation function:
test = np.random.random(input_shape)[np.newaxis,...] layer_outs = [func([test, 1.]) for func in functors] print layer_outs
Remember to set K.learning_phase() to 1 if any of the layers in your model include dropout or batch normalization to simulate training mode.
Optimizing the Process
A more efficient approach to evaluating layer outputs is to use a single function that returns the list of outputs for all layers:
from keras import backend as K functor = K.function([inp, K.learning_phase()], outputs)
This reduces the data transfer and computation overhead associated with individual function evaluations.
The above is the detailed content of How to Extract Activations from Specific Layers in a Keras Model?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
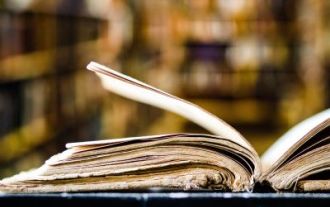
How to Use Python to Find the Zipf Distribution of a Text File
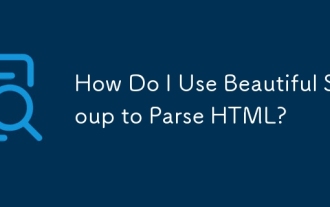
How Do I Use Beautiful Soup to Parse HTML?
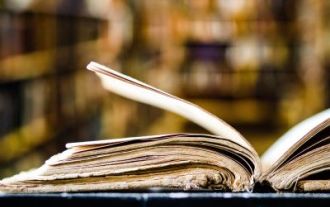
How to Work With PDF Documents Using Python
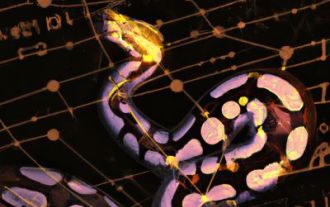
How to Cache Using Redis in Django Applications
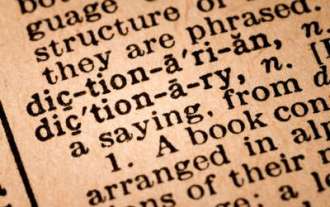
Introducing the Natural Language Toolkit (NLTK)
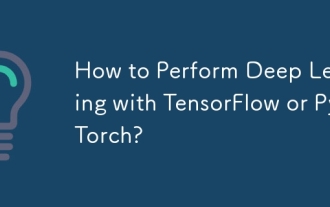
How to Perform Deep Learning with TensorFlow or PyTorch?
