


How to Implement an AJAX \'Load More Posts\' Feature in WordPress?
How to Implement an AJAX "Load More Posts" Feature in WordPress
This article addresses the issue of implementing an AJAX "Load More Posts" feature in WordPress, which allows users to dynamically load additional posts on a webpage without having to reload the entire page.
Problem Statement
The user encountered difficulties while attempting to implement this feature using various methods, including the one mentioned in the "Pagination on a Custom WP_Query Ajax" post.
Solution
The solution provided focuses on improving the code to ensure proper functionality. The key aspects include:
1. Server-Side Code
- In the template file, ensure that the initial post query returns the desired number of posts per page.
- In the functions file, create a function to handle the AJAX request and dynamically load more posts. The function should include pagination logic to retrieve the correct set of posts.
2. Client-Side Code (jQuery)
- On the client-side, bind an event listener to the "Load More" button.
- When the button is clicked, send an AJAX request to the server-side function using jQuery.
- Pass necessary parameters like the offset and number of posts per page.
- Append the returned HTML to the existing posts list.
3. Additional Considerations
- Handle the case of no more posts to load by disabling the "Load More" button.
- To create an "infinite load" effect, use window scrolling instead of button click events.
Code Implementation
Follow the provided code snippets to implement the solution:
functions.php:
/** * Handle AJAX "Load More" request */ function load_more_posts() { $offset = $_POST['offset']; $ppp = $_POST['ppp']; $args = [ 'post_type' => 'post', 'posts_per_page' => $ppp, 'offset' => $offset, // Other query parameters... ]; $query = new WP_Query($args); $html = ''; if ($query->have_posts()) { while ($query->have_posts()) { $query->the_post(); $html .= '<div>' . get_the_content() . '</div>'; } wp_reset_postdata(); } else { $html = 'No more posts to load'; } echo $html; exit(); } add_action('wp_ajax_load_more_posts', 'load_more_posts'); add_action('wp_ajax_nopriv_load_more_posts', 'load_more_posts');
script.js:
$(function() { var offset = 0; var ppp = 3; $('#load-more').on('click', function() { $.ajax({ type: 'POST', url: 'path/to/wp-admin/admin-ajax.php', data: { action: 'load_more_posts', offset: offset, ppp: ppp }, success: function(response) { $('#posts').append(response); offset += ppp; } }); // Prevent the button from triggering multiple AJAX requests $('#load-more').attr('disabled', true); }); });
Note: Replace 'path/to/wp-admin/admin-ajax.php' with the actual URL to your WordPress admin-ajax.php file.
Conclusion
By following these steps, you can effectively implement an AJAX "Load More Posts" feature for your WordPress site, allowing users to seamlessly browse through large amounts of content without having to refresh the entire page.
The above is the detailed content of How to Implement an AJAX \'Load More Posts\' Feature in WordPress?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










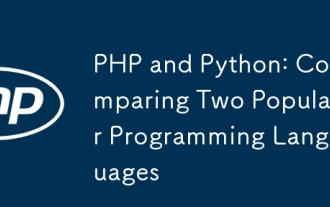
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
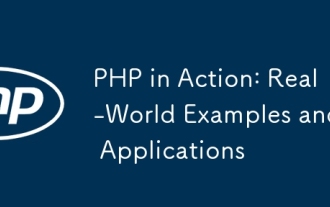
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
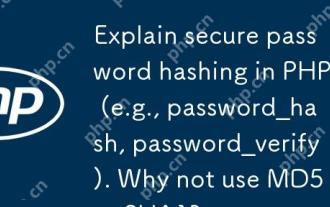
In PHP, password_hash and password_verify functions should be used to implement secure password hashing, and MD5 or SHA1 should not be used. 1) password_hash generates a hash containing salt values to enhance security. 2) Password_verify verify password and ensure security by comparing hash values. 3) MD5 and SHA1 are vulnerable and lack salt values, and are not suitable for modern password security.
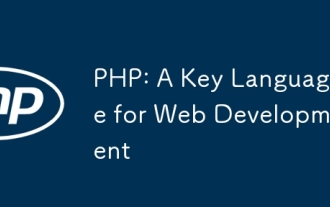
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
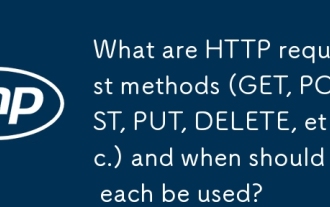
HTTP request methods include GET, POST, PUT and DELETE, which are used to obtain, submit, update and delete resources respectively. 1. The GET method is used to obtain resources and is suitable for read operations. 2. The POST method is used to submit data and is often used to create new resources. 3. The PUT method is used to update resources and is suitable for complete updates. 4. The DELETE method is used to delete resources and is suitable for deletion operations.
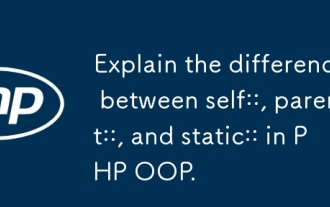
In PHPOOP, self:: refers to the current class, parent:: refers to the parent class, static:: is used for late static binding. 1.self:: is used for static method and constant calls, but does not support late static binding. 2.parent:: is used for subclasses to call parent class methods, and private methods cannot be accessed. 3.static:: supports late static binding, suitable for inheritance and polymorphism, but may affect the readability of the code.
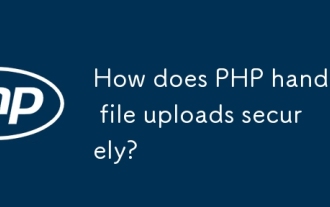
PHP handles file uploads through the $\_FILES variable. The methods to ensure security include: 1. Check upload errors, 2. Verify file type and size, 3. Prevent file overwriting, 4. Move files to a permanent storage location.
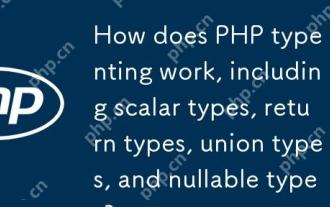
PHP type prompts to improve code quality and readability. 1) Scalar type tips: Since PHP7.0, basic data types are allowed to be specified in function parameters, such as int, float, etc. 2) Return type prompt: Ensure the consistency of the function return value type. 3) Union type prompt: Since PHP8.0, multiple types are allowed to be specified in function parameters or return values. 4) Nullable type prompt: Allows to include null values and handle functions that may return null values.
