


How to Retrieve HTML Element Style Values in JavaScript Across Browsers?
Retrieving HTML Element Style Values in JavaScript
For elements with styles defined through the style tag, as illustrated below:
<style> #box { width: 100px; } </style> <div>
To retrieve style values using JavaScript, the element.style property alone won't suffice, as it only provides inline or JavaScript-defined styles.
Computed Styles
We need to access the element's computed style, which represents the actual style applied to it after inheriting from parent elements and accounting for applied CSS rules. In JavaScript, we can obtain computed styles using two methods:
1. DOM Standard (Other Browsers):
document.defaultView.getComputedStyle(element, null).getPropertyValue(propertyName);
2. IE Specific:
element.currentStyle[propertyName];
However, IE uses camelCase property names (e.g., "font-Size") and may return sizes in non-pixel units, while the standard method uses hyphenated property names (e.g., "font-size") and always provides pixel values.
Cross-Browser Solution
To retrieve computed styles in a cross-browser compatible manner, use the following function:
function getStyle(element, property) { var value, defaultView = (element.ownerDocument || document).defaultView; // Sanitize property name for different browsers property = property.replace(/([A-Z])/g, "-").toLowerCase(); if (defaultView && defaultView.getComputedStyle) { return defaultView.getComputedStyle(element, null).getPropertyValue(property); } else if (element.currentStyle) { // Convert camelCase property name to hyphenated property = property.replace(/\-(\w)/g, function(str, letter) { return letter.toUpperCase(); }); value = element.currentStyle[property]; if (/^\d+(em|pt|%|ex)?$/i.test(value)) { return (function(value) { var oldLeft = element.style.left, oldRsLeft = element.runtimeStyle.left; element.runtimeStyle.left = element.currentStyle.left; element.style.left = value || 0; value = element.style.pixelLeft + "px"; element.style.left = oldLeft; element.runtimeStyle.left = oldRsLeft; return value; })(value); } return value; } }
The above is the detailed content of How to Retrieve HTML Element Style Values in JavaScript Across Browsers?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










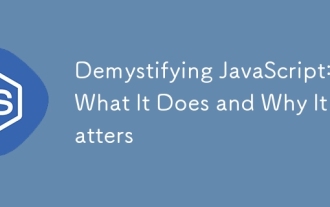
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
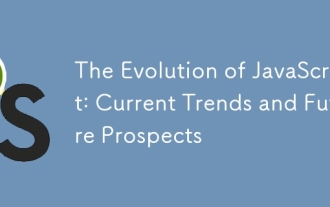
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
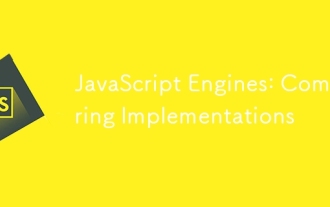
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
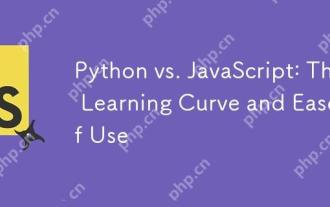
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
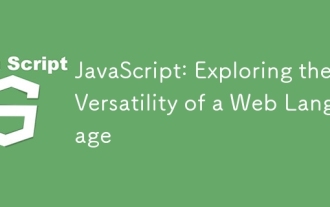
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
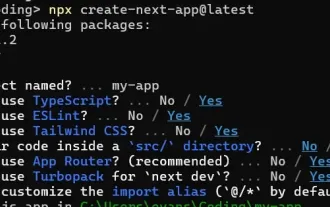
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
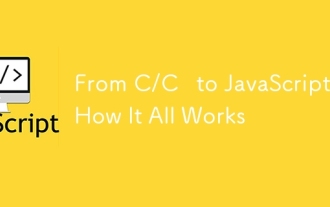
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
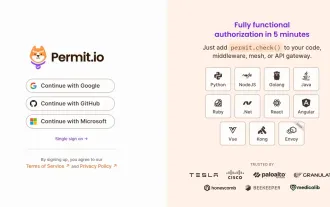
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
