How Can I Print the Textual Representation of a C Enum Value?
C : Printing Enum Value as Text
Enum types provide a convenient way to represent a set of constants with symbolic names. By default, enums are represented as integer values. However, in situations where you need to display the actual text associated with an enum value, the default behavior may not be suitable.
Let's consider an example:
enum Errors { ErrorA = 0, ErrorB, ErrorC, }; Errors anError = ErrorA; std::cout << anError; // Outputs "0"
In this example, we have an Errors enum with three possible values: ErrorA, ErrorB, and ErrorC, where ErrorA has a numerical value of 0. When we attempt to print the anError variable, it outputs "0" instead of the desired "ErrorA".
To solve this issue without resorting to if/switch statements, several methods can be employed:
1. Using a Map:
#include <map> #include <string_view> // Define a map to associate enum values with their string representations std::map<Errors, std::string_view> errorStrings = { {ErrorA, "ErrorA"}, {ErrorB, "ErrorB"}, {ErrorC, "ErrorC"}, }; // Overload the `<<` operator to print the string representation std::ostream& operator<<(std::ostream& out, const Errors value) { out << errorStrings[value]; return out; }
With this method, the overloaded << operator looks up the string representation associated with an enum value in the errorStrings map and outputs it.
2. Using an Array of Structures:
#include <string_view> // Define a struct to store enum values and string representations struct MapEntry { Errors value; std::string_view str; }; // Define an array of structures MapEntry entries[] = { {ErrorA, "ErrorA"}, {ErrorB, "ErrorB"}, {ErrorC, "ErrorC"}, }; // Overload the `<<` operator to perform a linear search and print the string representation std::ostream& operator<<(std::ostream& out, const Errors value) { for (const MapEntry& entry : entries) { if (entry.value == value) { out << entry.str; break; } } return out; }
This method uses an array of structures to store the enum values and their string representations. A linear search is performed to find the matching string for the specified enum value.
3. Using switch/case:
#include <string> // Overload the `<<` operator to print the string representation using `switch/case` std::ostream& operator<<(std::ostream& out, const Errors value) { switch (value) { case ErrorA: out << "ErrorA"; break; case ErrorB: out << "ErrorB"; break; case ErrorC: out << "ErrorC"; break; } return out; }
In this method, the overloaded << operator uses a switch/case statement to directly print the appropriate string representation for the given enum value.
The above is the detailed content of How Can I Print the Textual Representation of a C Enum Value?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










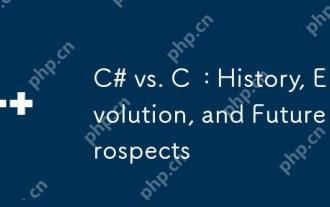
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
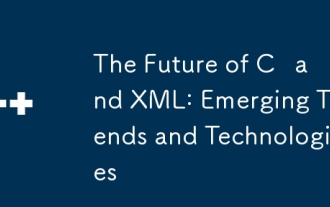
The future development trends of C and XML are: 1) C will introduce new features such as modules, concepts and coroutines through the C 20 and C 23 standards to improve programming efficiency and security; 2) XML will continue to occupy an important position in data exchange and configuration files, but will face the challenges of JSON and YAML, and will develop in a more concise and easy-to-parse direction, such as the improvements of XMLSchema1.1 and XPath3.1.
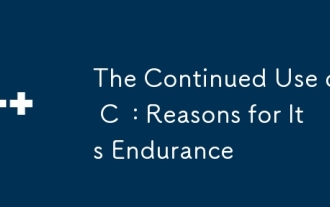
C Reasons for continuous use include its high performance, wide application and evolving characteristics. 1) High-efficiency performance: C performs excellently in system programming and high-performance computing by directly manipulating memory and hardware. 2) Widely used: shine in the fields of game development, embedded systems, etc. 3) Continuous evolution: Since its release in 1983, C has continued to add new features to maintain its competitiveness.
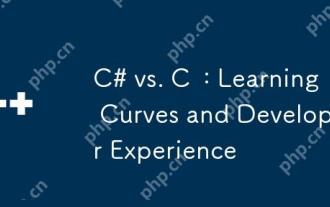
There are significant differences in the learning curves of C# and C and developer experience. 1) The learning curve of C# is relatively flat and is suitable for rapid development and enterprise-level applications. 2) The learning curve of C is steep and is suitable for high-performance and low-level control scenarios.
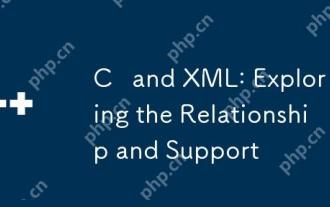
C interacts with XML through third-party libraries (such as TinyXML, Pugixml, Xerces-C). 1) Use the library to parse XML files and convert them into C-processable data structures. 2) When generating XML, convert the C data structure to XML format. 3) In practical applications, XML is often used for configuration files and data exchange to improve development efficiency.
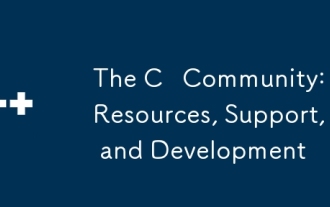
C Learners and developers can get resources and support from StackOverflow, Reddit's r/cpp community, Coursera and edX courses, open source projects on GitHub, professional consulting services, and CppCon. 1. StackOverflow provides answers to technical questions; 2. Reddit's r/cpp community shares the latest news; 3. Coursera and edX provide formal C courses; 4. Open source projects on GitHub such as LLVM and Boost improve skills; 5. Professional consulting services such as JetBrains and Perforce provide technical support; 6. CppCon and other conferences help careers
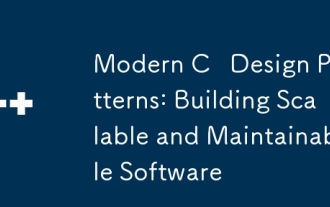
The modern C design model uses new features of C 11 and beyond to help build more flexible and efficient software. 1) Use lambda expressions and std::function to simplify observer pattern. 2) Optimize performance through mobile semantics and perfect forwarding. 3) Intelligent pointers ensure type safety and resource management.
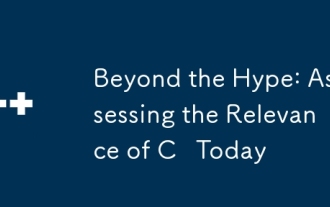
C still has important relevance in modern programming. 1) High performance and direct hardware operation capabilities make it the first choice in the fields of game development, embedded systems and high-performance computing. 2) Rich programming paradigms and modern features such as smart pointers and template programming enhance its flexibility and efficiency. Although the learning curve is steep, its powerful capabilities make it still important in today's programming ecosystem.
