


How Can I Detect 'Shift Enter' to Insert a New Line in a Textarea Instead of Submitting a Form?
Nov 30, 2024 am 09:25 AMDetecting "Shift Enter" and Inserting a New Line in Textarea
By default, pressing the "Enter" key inside a textarea submits the form. However, you want to retain this functionality while also allowing users to create a new line by pressing the "Shift" "Enter" key combination. Here's how to achieve this in JavaScript:
If you control the script that makes "Enter" submit the form, simply modify the code to check if the "Shift" key is not pressed. For instance, change this:
if (evt.keyCode == 13) { form.submit(); }
... to this:
if (evt.keyCode == 13 && !evt.shiftKey) { form.submit(); }
However, if you don't have access to the original code, you can use the following script to achieve the desired behavior, even when the cursor is not at the end of the text:
function handleEnter(evt) { if (evt.keyCode == 13 && evt.shiftKey) { if (evt.type == "keypress") { pasteIntoInput(this, "\n"); } evt.preventDefault(); } } // Handle both keydown and keypress for Opera as it suppresses default key action in keypress $("#your_textarea_id").keydown(handleEnter).keypress(handleEnter);
This script checks for the "Shift" "Enter" key combination. When detected, it inserts a newline character ("n") into the textarea using the pasteIntoInput function and prevents the default form submission.
The above is the detailed content of How Can I Detect 'Shift Enter' to Insert a New Line in a Textarea Instead of Submitting a Form?. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
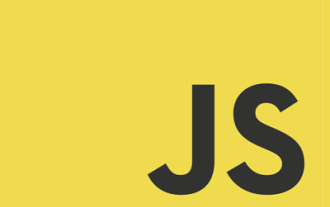
Create a JavaScript Contact Form With the Smart Forms Framework
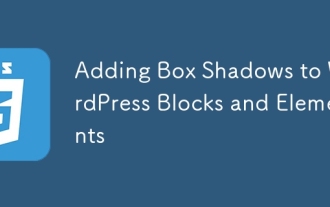
Adding Box Shadows to WordPress Blocks and Elements
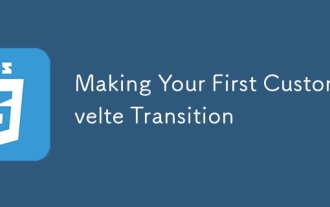
Making Your First Custom Svelte Transition
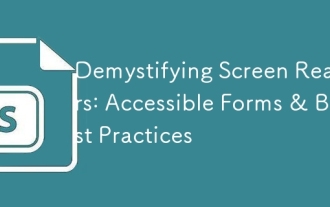
Demystifying Screen Readers: Accessible Forms & Best Practices
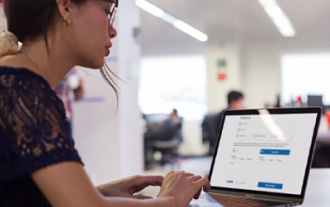
Comparing the 5 Best PHP Form Builders (And 3 Free Scripts)
