How Can I Integrate Stanford Parser with NLTK in Python?
Integrating Stanford Parser into NLTK with Python
Can Stanford Parser be leveraged within NLTK?
Yes, it is possible to utilize Stanford Parser within the NLTK framework using Python. The following Python code snippet demonstrates how to achieve this:
import os from nltk.parse import stanford # Specify paths to Stanford Parser and models os.environ['STANFORD_PARSER'] = '/path/to/standford/jars' os.environ['STANFORD_MODELS'] = '/path/to/standford/jars' # Initialize the Stanford Parser parser = stanford.StanfordParser(model_path="/location/of/the/englishPCFG.ser.gz") # Parse a list of sample sentences sentences = parser.raw_parse_sents(("Hello, My name is Melroy.", "What is your name?")) print sentences # Visualize the dependency tree for line in sentences: for sentence in line: sentence.draw()
This example showcases the parsed dependency trees for the provided sentences:
[Tree('ROOT', [Tree('S', [Tree('INTJ', [Tree('UH', ['Hello'])]), Tree(',', [',']), Tree('NP', [Tree('PRP$', ['My']), Tree('NN', ['name'])]), Tree('VP', [Tree('VBZ', ['is']), Tree('ADJP', [Tree('JJ', ['Melroy'])])]), Tree('.', ['.'])])]), Tree('ROOT', [Tree('SBARQ', [Tree('WHNP', [Tree('WP', ['What'])]), Tree('SQ', [Tree('VBZ', ['is']), Tree('NP', [Tree('PRP$', ['your']), Tree('NN', ['name'])])]), Tree('.', ['?'])])])}
Key Notes:
- In this example, both the parser and model jars reside in the same directory.
- The Stanford Parser's filename is stanford-parser.jar.
- The Stanford model's filename is stanford-parser-x.x.x-models.jar.
- The englishPCFG.ser.gz file is located within the models.jar file and needs to be extracted for use.
- Java JRE 1.8 (Java Development Kit 8) is required.
Installation Instructions:
Using NLTK v3 Installer:
- Download and install NLTK v3.
- Use the NLTK downloader:
import nltk nltk.download()
Manual Installation:
- Download and install NLTK v3.
- Download the latest Stanford Parser version.
- Extract the stanford-parser-3.x.x-models.jar and stanford-parser.jar files.
- Place these files in a designated 'jars' folder and set the STANFORD_PARSER and STANFORD_MODELS environment variables to point to this folder.
- Extract the englishPCFG.ser.gz file from the models.jar file and note its location.
- Create a StanfordParser instance using the specified model path.
The above is the detailed content of How Can I Integrate Stanford Parser with NLTK in Python?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










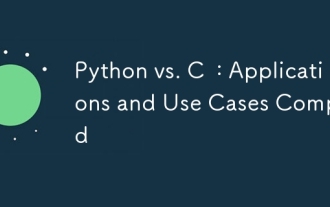
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
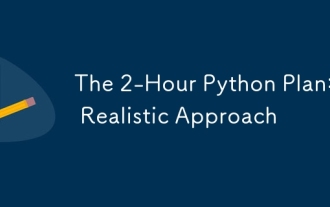
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
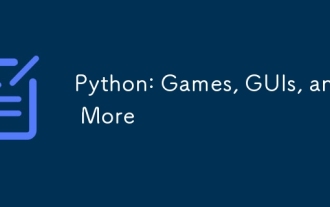
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
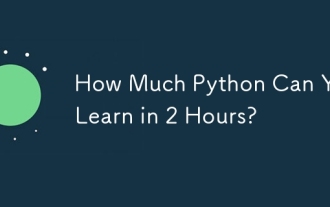
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
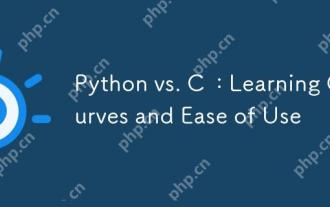
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
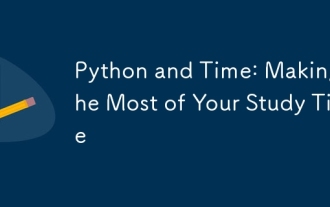
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
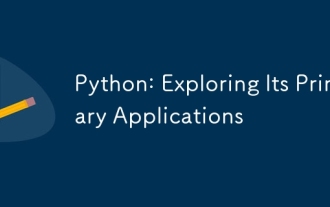
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
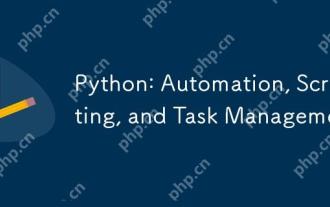
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
