


How Can I Efficiently Sort a Globbed File Array by Last Modified Datetime in PHP?
Globally Sorted File Arrays by Last Modified Datetime Stamp
Sorting files based on their last modified datetime stamp is a common task when managing and organizing file systems. The glob() function provides a quick method to retrieve files matching a specified pattern, but what if you want to further sort the resulting array by date?
Custom Sorting Approach
One approach is to iterate through the initial array and create a new array with the sorted files. While this works, it can be inefficient for larger arrays. A more efficient method exists.
Utilizing the Usort Function
PHP offers the usort() function, which sorts an array using a user-defined comparison function. This allows you to specify how the elements should be compared and ordered. In our case, we can use filemtime() to retrieve the last modified timestamp for each element and then sort the array based on these timestamps.
The following code snippet demonstrates how to sort an array of files by last modified datetime stamp using usort():
// Retrieve an array of files using glob() $myarray = glob("*.*"); // Define a comparison function for usort() $compare = function($a, $b) { return filemtime($a) - filemtime($b); }; // Sort the array using usort() usort($myarray, $compare); // Now the $myarray contains files sorted by last modified timestamp
Note that this approach requires PHP version 5.3 or later, as create_function() is deprecated in PHP 7.2.0 and above. If you are using an older version of PHP, you can still achieve similar functionality using an anonymous function or a closure.
By utilizing usort() and filemtime(), you can efficiently sort an array of files by their last modified datetime stamp, providing a more organized and manageable file list.
The above is the detailed content of How Can I Efficiently Sort a Globbed File Array by Last Modified Datetime in PHP?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










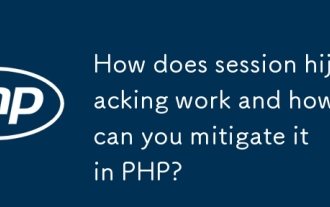
Session hijacking can be achieved through the following steps: 1. Obtain the session ID, 2. Use the session ID, 3. Keep the session active. The methods to prevent session hijacking in PHP include: 1. Use the session_regenerate_id() function to regenerate the session ID, 2. Store session data through the database, 3. Ensure that all session data is transmitted through HTTPS.
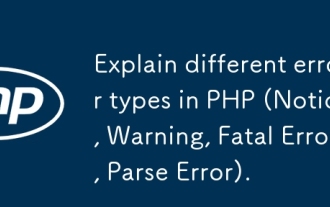
There are four main error types in PHP: 1.Notice: the slightest, will not interrupt the program, such as accessing undefined variables; 2. Warning: serious than Notice, will not terminate the program, such as containing no files; 3. FatalError: the most serious, will terminate the program, such as calling no function; 4. ParseError: syntax error, will prevent the program from being executed, such as forgetting to add the end tag.
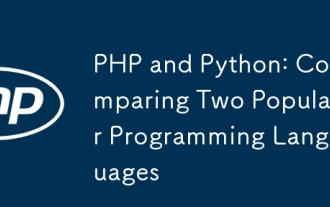
PHP and Python each have their own advantages, and choose according to project requirements. 1.PHP is suitable for web development, especially for rapid development and maintenance of websites. 2. Python is suitable for data science, machine learning and artificial intelligence, with concise syntax and suitable for beginners.
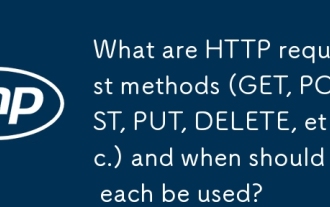
HTTP request methods include GET, POST, PUT and DELETE, which are used to obtain, submit, update and delete resources respectively. 1. The GET method is used to obtain resources and is suitable for read operations. 2. The POST method is used to submit data and is often used to create new resources. 3. The PUT method is used to update resources and is suitable for complete updates. 4. The DELETE method is used to delete resources and is suitable for deletion operations.
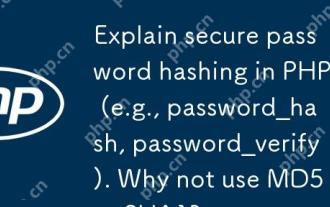
In PHP, password_hash and password_verify functions should be used to implement secure password hashing, and MD5 or SHA1 should not be used. 1) password_hash generates a hash containing salt values to enhance security. 2) Password_verify verify password and ensure security by comparing hash values. 3) MD5 and SHA1 are vulnerable and lack salt values, and are not suitable for modern password security.
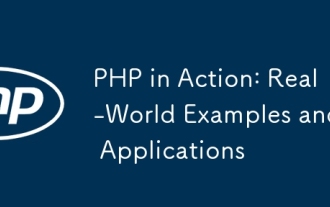
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
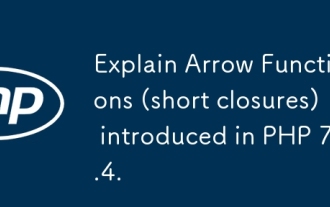
The arrow function was introduced in PHP7.4 and is a simplified form of short closures. 1) They are defined using the => operator, omitting function and use keywords. 2) The arrow function automatically captures the current scope variable without the use keyword. 3) They are often used in callback functions and short calculations to improve code simplicity and readability.
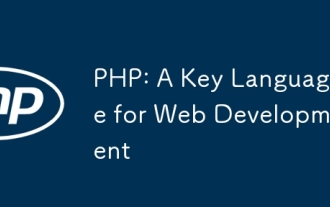
PHP is a scripting language widely used on the server side, especially suitable for web development. 1.PHP can embed HTML, process HTTP requests and responses, and supports a variety of databases. 2.PHP is used to generate dynamic web content, process form data, access databases, etc., with strong community support and open source resources. 3. PHP is an interpreted language, and the execution process includes lexical analysis, grammatical analysis, compilation and execution. 4.PHP can be combined with MySQL for advanced applications such as user registration systems. 5. When debugging PHP, you can use functions such as error_reporting() and var_dump(). 6. Optimize PHP code to use caching mechanisms, optimize database queries and use built-in functions. 7
