Why Doesn\'t My JPanel Update After Adding New Images in My Puzzle Game?
JPanel Update Issue in Puzzle Game
In your puzzle game, you encounter an issue where JPanel doesn't update despite adding updated images. Let's delve into the problem and provide solutions.
The issue arises because after modifying the images in the Img[], the addComponents method is called to add the updated images to the JPanel. However, this method only adds the components without invoking a panel repaint.
To ensure that the JPanel updates correctly, you need to explicitly call the repaint and validate methods after modifying the components. The repaint method initiates the rendering process, while validate ensures that the layout is up-to-date and applied to the components.
Updated Code:
public void addComponents(Img[] im){ this.removeAll(); for(int i=0; i<16; i++){ im[i].addActionListener(this); im[i].setPreferredSize(new Dimension(53,53)); add(im[i]); } this.validate(); this.repaint(); }
Additional Considerations:
In your code, you use a JButton as the Img class, which is not ideal for drawing images. It's recommended to extend the JPanel class for drawing custom components. Alternatively, you can use JLabel to display images.
Also, when shuffling the images, it's possible that the correct image may appear in place. To avoid this, you can shuffle the images randomly and check if they are in their correct places before adding them to the JPanel.
Alternative Approach:
Instead of using separate images, you can also slice a single image into tiles and add them to the JPanel. This eliminates the need to manage multiple images and simplifies the updating process.
The above is the detailed content of Why Doesn\'t My JPanel Update After Adding New Images in My Puzzle Game?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


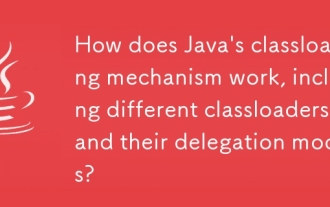
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa
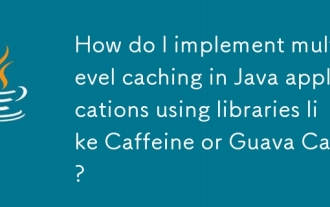
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra
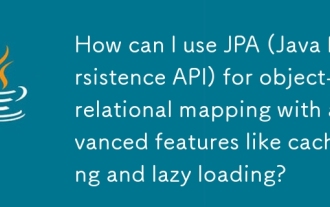
The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
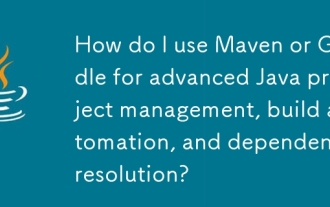
The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
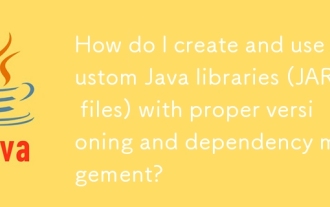
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
