


How Can I Efficiently Traverse a JSON Object Tree in Pure JavaScript?
Traversal of JSON Object Tree in JavaScript without External Libraries
Traversing a JSON object tree can be a common task when working with complex data structures. While JavaScript provides a rich library of functions for working with objects, there is no dedicated library for traversing object trees. This may seem like a straightforward task, but it often leads to reinventing the wheel.
Unlike XML, which offers various DOM-based approaches for traversing trees, JavaScript lacks similar mechanisms for JSON objects. This article presents an efficient and simple solution to traverse JSON object trees using pure JavaScript functions.
Custom Recursive Traversal Function
The solution involves creating a custom recursive function that traverses the object tree. The function traverse() takes two parameters:
- o: The JSON object to traverse
- func: A callback function that processes each node in the tree
The function iterates through each property and its value in the object. For each property-value pair, it calls the callback function to process the data. If the value is another object and not null, the function recursively calls itself to traverse the child object.
Example Usage
To demonstrate the usage, let's consider the following JSON object:
var o = { foo:"bar", arr:[1,2,3], subo: { foo2:"bar2" } };
We define a process() function to log the key and value of each node:
function process(key,value) { console.log(key + " : "+value); }
Now, we can traverse the object tree using traverse():
traverse(o,process);
This will output the following:
foo : bar arr : 1 arr : 2 arr : 3 subo : [object Object] foo2 : bar2
Note that the output includes the subobject's key but not its properties. This is because the traverse() function does not recursively traverse the subobject. To achieve full-depth traversal, one would need to modify the code accordingly.
The above is the detailed content of How Can I Efficiently Traverse a JSON Object Tree in Pure JavaScript?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










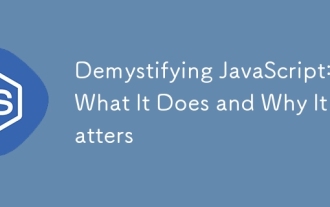
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
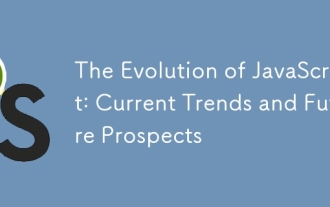
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
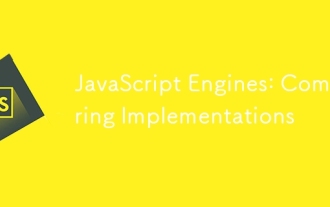
Different JavaScript engines have different effects when parsing and executing JavaScript code, because the implementation principles and optimization strategies of each engine differ. 1. Lexical analysis: convert source code into lexical unit. 2. Grammar analysis: Generate an abstract syntax tree. 3. Optimization and compilation: Generate machine code through the JIT compiler. 4. Execute: Run the machine code. V8 engine optimizes through instant compilation and hidden class, SpiderMonkey uses a type inference system, resulting in different performance performance on the same code.
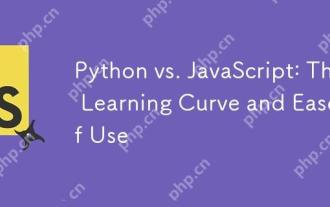
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
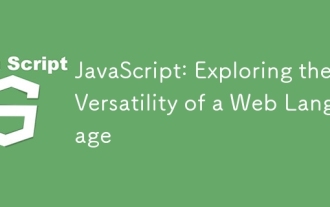
JavaScript is the core language of modern web development and is widely used for its diversity and flexibility. 1) Front-end development: build dynamic web pages and single-page applications through DOM operations and modern frameworks (such as React, Vue.js, Angular). 2) Server-side development: Node.js uses a non-blocking I/O model to handle high concurrency and real-time applications. 3) Mobile and desktop application development: cross-platform development is realized through ReactNative and Electron to improve development efficiency.
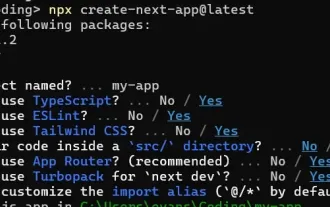
This article demonstrates frontend integration with a backend secured by Permit, building a functional EdTech SaaS application using Next.js. The frontend fetches user permissions to control UI visibility and ensures API requests adhere to role-base
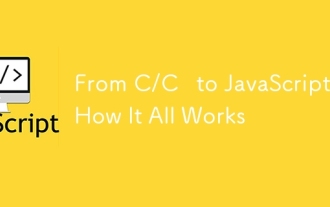
The shift from C/C to JavaScript requires adapting to dynamic typing, garbage collection and asynchronous programming. 1) C/C is a statically typed language that requires manual memory management, while JavaScript is dynamically typed and garbage collection is automatically processed. 2) C/C needs to be compiled into machine code, while JavaScript is an interpreted language. 3) JavaScript introduces concepts such as closures, prototype chains and Promise, which enhances flexibility and asynchronous programming capabilities.
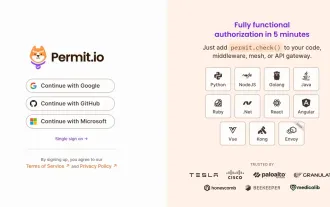
I built a functional multi-tenant SaaS application (an EdTech app) with your everyday tech tool and you can do the same. First, what’s a multi-tenant SaaS application? Multi-tenant SaaS applications let you serve multiple customers from a sing
