


How to Simultaneously Spawn Multiple Circle Instances in a Pygame Game?
Spawning Multiple Instances of the Same Object Simultaneously in Python
In this programming inquiry, a novice coder seeking guidance in creating a game using Python's Pygame engine encounters a challenge. The objective is to spawn multiple instances of circles, granting points upon their clicking. However, the developer encounters an issue where subsequent circles override preceding ones.
Understanding the Problem
The issue arises due to the nature of the game loop. Functions like sleep() and pygame.time.wait() do not effectively control time within the application's loop. As the game continues, the previous circles are cleared from the screen before the new ones can be drawn.
Solution Options
To address this problem, there are two primary solution options:
1. Time-Based Spawning
Use pygame.time.get_ticks() to measure the elapsed time in the loop. Define a time interval for object creation and spawn a new object when the specified time has elapsed. This method allows for precise control over the timing of object spawning.
2. Pygame Event Module
Utilize pygame.time.set_timer() to create a custom event in the event queue, triggering the object creation. This approach provides greater flexibility in scheduling object spawns with specific time intervals.
Applying the Solutions
Time-Based Spawning Example:
import pygame, random pygame.init() window = pygame.display.set_mode((300, 300)) class Circle: def __init__(self): ... object_list = [] time_interval = 200 # 200 milliseconds == 0.2 seconds next_object_time = 0 run = True clock = pygame.time.Clock() while run: clock.tick(60) for event in pygame.event.get(): if event.type == pygame.QUIT: run = False current_time = pygame.time.get_ticks() if current_time > next_object_time: next_object_time += time_interval object_list.append(Circle()) window.fill(0) for circle in object_list[:]: ...
Pygame Event Module Example:
import pygame, random pygame.init() window = pygame.display.set_mode((300, 300)) class Circle: def __init__(self): ... object_list = [] time_interval = 200 # 200 milliseconds == 0.2 seconds timer_event = pygame.USEREVENT+1 pygame.time.set_timer(timer_event, time_interval) run = True clock = pygame.time.Clock() while run: clock.tick(60) for event in pygame.event.get(): if event.type == pygame.QUIT: run = False elif event.type == timer_event: object_list.append(Circle())
By implementing one of these solutions, you can effectively spawn multiple instances of the same object concurrently in your Pygame game, allowing for a more dynamic and engaging gameplay experience.
The above is the detailed content of How to Simultaneously Spawn Multiple Circle Instances in a Pygame Game?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










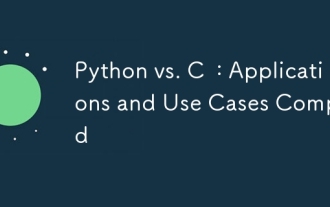
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
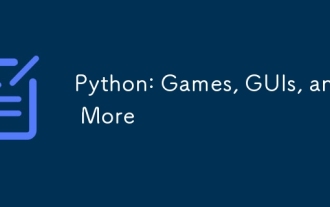
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
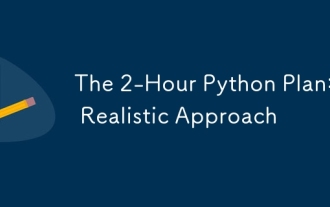
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
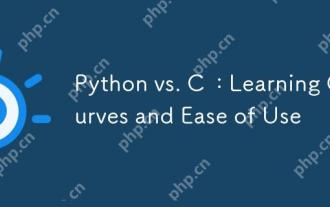
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
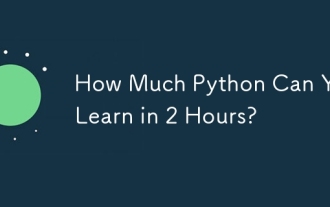
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
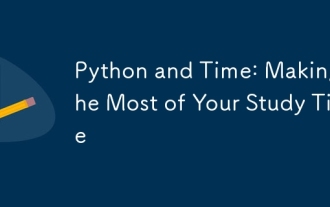
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
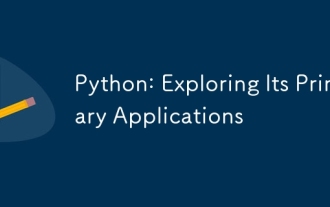
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
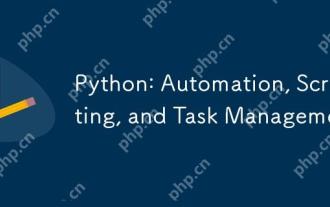
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
