


How to Programmatically Generate XPath Expressions from an XML Document in Java?
How to Generate/Get XPath from XML in Java
Introduction
This article will provide guidance on extracting XPath expressions from an XML document in Java. It covers the process of traversing XML nodes, checking for attribute existence, and generating XPath strings accordingly.
Goal
Our objective is to generate XPath expressions from the following XML document:
<root> <elemA>one</elemA> <elemA attribute1='first' attribute2='second'>two</elemA> <elemB>three</elemB> <elemA>four</elemA> <elemC> <elemB>five</elemB> </elemC> </root>
and obtain the following XPath expressions:
//root[1]/elemA[1]='one' //root[1]/elemA[2]='two' //root[1]/elemA[2][@attribute1='first'] //root[1]/elemA[2][@attribute2='second'] //root[1]/elemB[1]='three' //root[1]/elemA[3]='four' //root[1]/elemC[1]/elemB[1]='five'
Implementation
1. Traverse the XML Document:
Use a Java XML parsing library, such as JDOM or SAX, to navigate through the XML nodes.
2. Check for Attribute Existence:
For each node, check if it has any attributes. If not, proceed with XPath generation.
3. Generate XPath for Nodes with Attributes:
For nodes with attributes, create XPath expressions for both the node and each attribute.
4. Combine XPath Expressions:
Concatenate the XPath expressions generated for the node and attributes to create the final XPath string. For example:
String xpath = "//root[1]/elemA[1]='" + nodeValue + "'";
5. Handle Special Cases:
Consider special cases such as spaces in XPath expressions or the need to enclose attribute values in single quotes.
Example
The following code snippet demonstrates the implementation in Java:
import java.io.File; import java.io.IOException; import java.util.ArrayList; import java.util.List; import javax.xml.parsers.DocumentBuilder; import javax.xml.parsers.DocumentBuilderFactory; import javax.xml.parsers.ParserConfigurationException; import org.w3c.dom.Document; import org.w3c.dom.Element; import org.w3c.dom.NamedNodeMap; import org.w3c.dom.Node; import org.w3c.dom.NodeList; import org.xml.sax.SAXException; public class GenerateXPath { public static void main(String[] args) throws SAXException, IOException, ParserConfigurationException { // Read the XML file File xmlFile = new File("path_to_xml.xml"); DocumentBuilderFactory dbFactory = DocumentBuilderFactory.newInstance(); DocumentBuilder dBuilder = dbFactory.newDocumentBuilder(); Document doc = dBuilder.parse(xmlFile); // Traverse the XML nodes NodeList nodes = doc.getElementsByTagName("*"); for (int i = 0; i < nodes.getLength(); i++) { Node node = nodes.item(i); // Check for attribute existence if (node.getAttributes().getLength() == 0) { // Generate XPath for nodes without attributes String xpath = generateXPath(node); System.out.println(xpath); } else { // Generate XPath for nodes with attributes xpath = generateXPathWithAttributes(node); System.out.println(xpath); } } } private static String generateXPath(Node node) { String xpath = ""; // Append tag name xpath += "/" + node.getNodeName(); // Append position index NodeList siblings = node.getParentNode().getChildNodes(); int position = 1; for (int i = 0; i < siblings.getLength(); i++) { if (siblings.item(i).getNodeName().equals(node.getNodeName())) { position++; } } xpath += "[" + position + "]"; return xpath; } private static String generateXPathWithAttributes(Node node) { List<String> xpathParts = new ArrayList<>(); // Append tag name xpathParts.add("/" + node.getNodeName()); // Append position index NodeList siblings = node.getParentNode().getChildNodes(); int position = 1; for (int i = 0; i < siblings.getLength(); i++) { if (siblings.item(i).getNodeName().equals(node.getNodeName())) { position++; } } xpathParts.add("[" + position + "]"); // Append attributes NamedNodeMap attributes = node.getAttributes(); for (int i = 0; i < attributes.getLength(); i++) { Node attribute = attributes.item(i); xpathParts.add("[@" + attribute.getNodeName() + "='" + attribute.getNodeValue() + "']"); } return String.join("", xpathParts); } }
Conclusion
Following the steps outlined above, it is possible to programmatically generate XPath expressions for XML documents in Java, providing an automated approach for extracting data from XML content.
The above is the detailed content of How to Programmatically Generate XPath Expressions from an XML Document in Java?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










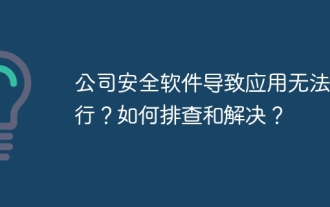
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
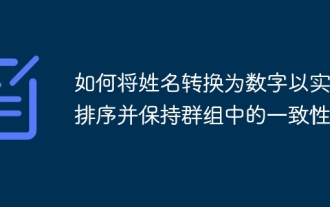
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
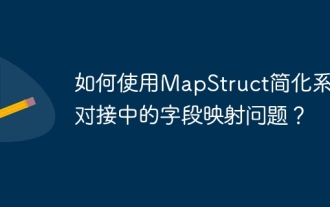
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
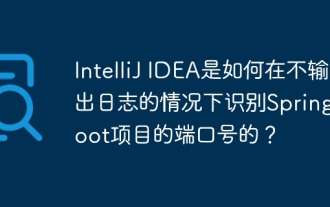
Start Spring using IntelliJIDEAUltimate version...
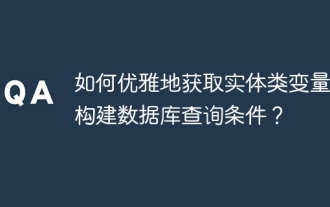
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
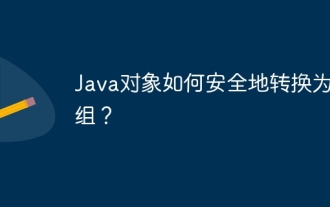
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
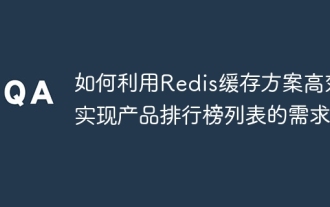
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
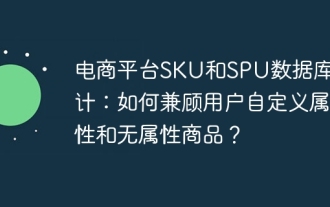
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
