How to Preserve POST Body Parameters in Java Servlets for Multiple Reads?
How to Preserve POST Body Parameters in Http Servlet Request
When working with HTTP requests in Java servlets, it's essential to consider the impact of accessing request parameters on further processing in the filter chain. By default, reading parameters from the POST request body consumes them, making them unavailable to subsequent filters or handlers.
The Problem: Consuming POST Body Parameters
In Java servlets, the request body parameters are accessible through request.getParameter(). However, this method consumes the inputStream and therefore subsequent attempts to retrieve the parameters will fail. This behavior is particularly problematic for filters that need to access the parameters before they are consumed by downstream handlers.
Solution: Extending HttpServletRequestWrapper
To address this issue, we can extend HttpServletRequestWrapper to create a custom request implementation that caches the request body. This allows us to read the body multiple times without consuming it.
Here's an example implementation:
public class MultiReadHttpServletRequest extends HttpServletRequestWrapper { private ByteArrayOutputStream cachedBytes; public MultiReadHttpServletRequest(HttpServletRequest request) { super(request); } @Override public ServletInputStream getInputStream() throws IOException { if (cachedBytes == null) { cacheInputStream(); } return new CachedServletInputStream(cachedBytes.toByteArray()); } private void cacheInputStream() throws IOException { cachedBytes = new ByteArrayOutputStream(); IOUtils.copy(super.getInputStream(), cachedBytes); } private static class CachedServletInputStream extends ServletInputStream { private final ByteArrayInputStream buffer; public CachedServletInputStream(byte[] contents) { this.buffer = new ByteArrayInputStream(contents); } @Override public int read() { return buffer.read(); } // Implement other methods required by ServletInputStream interface, such as isFinished, isReady, setReadListener, etc. } }
Usage:
To preserve POST body parameters, we can wrap the original request in our MultiReadHttpServletRequest before passing it through the filter chain:
public class MyFilter implements Filter { @Override public void doFilter(ServletRequest request, ServletResponse response, FilterChain chain) throws IOException, ServletException { // Wrap the request to enable multiple reads of the request body MultiReadHttpServletRequest multiReadRequest = new MultiReadHttpServletRequest((HttpServletRequest) request); // Read the request body for our own processing doMyThing(multiReadRequest.getInputStream()); // Continue the filter chain with the wrapped request, allowing subsequent handlers to access the cached request body chain.doFilter(multiReadRequest, response); } }
By using this approach, we can access POST body parameters multiple times without having to worry about consuming them prematurely.
The above is the detailed content of How to Preserve POST Body Parameters in Java Servlets for Multiple Reads?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










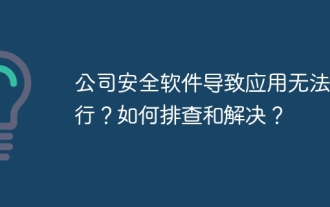
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
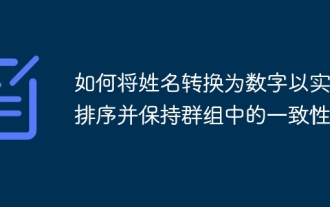
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
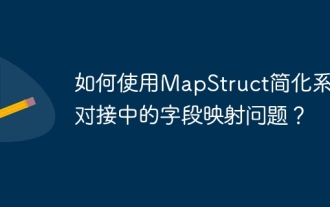
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
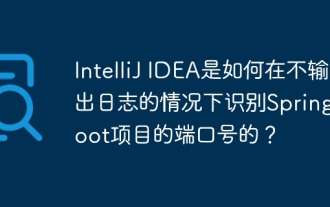
Start Spring using IntelliJIDEAUltimate version...
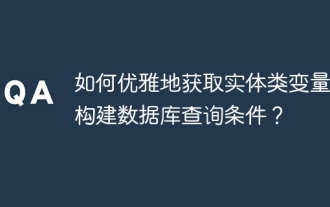
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
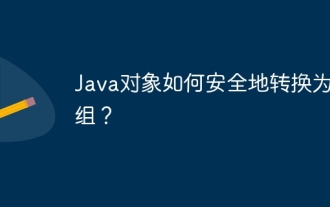
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
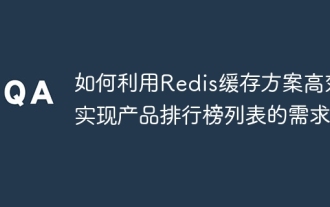
How does the Redis caching solution realize the requirements of product ranking list? During the development process, we often need to deal with the requirements of rankings, such as displaying a...
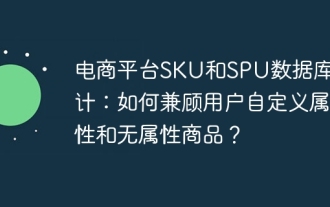
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
