How Can I Cancel a Long-Polling HTTP Request in Go?
Cancelling a Long-Polling Request in Golang
When implementing a client-side long-poll using http.Client, it is often desirable to have the ability to cancel the request prematurely. This could be achieved by manually calling resp.Body.Close() from a separate goroutine, but it is not a standard and elegant solution.
Cancellation Using Request Context
A more idiomatic way to cancel an HTTP request in Golang is by using the request context. By passing a context with a deadline or a cancel function, the request can be cancelled from the client side. The following code demonstrates how to use the request context to cancel a long-polling request:
import ( "context" "net/http" ) func main() { // Create a new HTTP client with a custom transport. client := &http.Client{ Transport: &http.Transport{ // Set a timeout for the request. Dial: (&net.Dialer{Timeout: 5 * time.Second}).Dial, TLSHandshakeTimeout: 5 * time.Second, }, } // Create a new request with a context that can be cancelled. ctx, cancel := context.WithTimeout(context.Background(), 10*time.Second) req, err := http.NewRequest("POST", "http://example.com", nil) if err != nil { panic(err) } req = req.WithContext(ctx) // Start the request in a goroutine and cancel it after 5 seconds. go func() { _, err := client.Do(req) if err != nil { // Handle the canceled error. } }() time.Sleep(5 * time.Second) cancel() }
When the cancel() function is called, the request will be cancelled and the Dial and TLSHandshakeTimeout specified in the transport will be respected.
The above is the detailed content of How Can I Cancel a Long-Polling HTTP Request in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










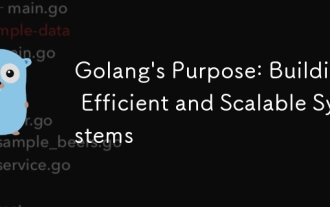
Go language performs well in building efficient and scalable systems. Its advantages include: 1. High performance: compiled into machine code, fast running speed; 2. Concurrent programming: simplify multitasking through goroutines and channels; 3. Simplicity: concise syntax, reducing learning and maintenance costs; 4. Cross-platform: supports cross-platform compilation, easy deployment.
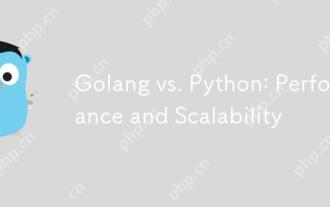
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
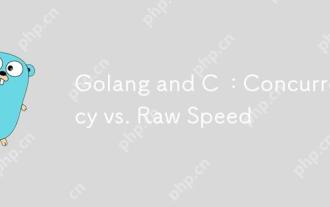
Golang is better than C in concurrency, while C is better than Golang in raw speed. 1) Golang achieves efficient concurrency through goroutine and channel, which is suitable for handling a large number of concurrent tasks. 2)C Through compiler optimization and standard library, it provides high performance close to hardware, suitable for applications that require extreme optimization.
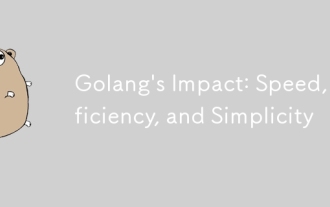
Goimpactsdevelopmentpositivelythroughspeed,efficiency,andsimplicity.1)Speed:Gocompilesquicklyandrunsefficiently,idealforlargeprojects.2)Efficiency:Itscomprehensivestandardlibraryreducesexternaldependencies,enhancingdevelopmentefficiency.3)Simplicity:
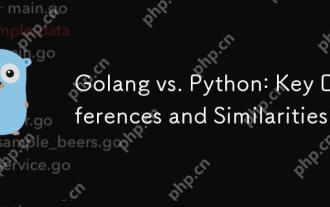
Golang and Python each have their own advantages: Golang is suitable for high performance and concurrent programming, while Python is suitable for data science and web development. Golang is known for its concurrency model and efficient performance, while Python is known for its concise syntax and rich library ecosystem.
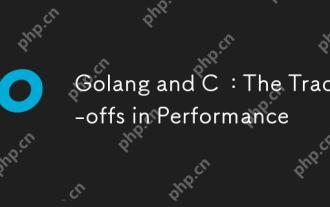
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
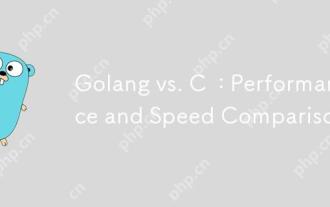
Golang is suitable for rapid development and concurrent scenarios, and C is suitable for scenarios where extreme performance and low-level control are required. 1) Golang improves performance through garbage collection and concurrency mechanisms, and is suitable for high-concurrency Web service development. 2) C achieves the ultimate performance through manual memory management and compiler optimization, and is suitable for embedded system development.
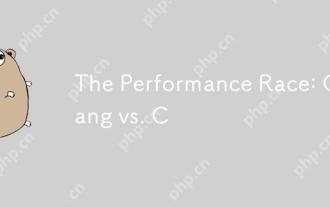
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
